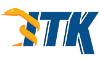 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkRandomImageSource_h
29 #define itkRandomImageSource_h
54 template <
typename TOutputImage>
92 static constexpr
unsigned int OutputImageDimension = TOutputImage::ImageDimension;
126 itkSetClampMacro(Min,
136 itkSetClampMacro(Max,
148 PrintSelf(std::ostream & os,
Indent indent)
const override;
154 GenerateOutputInformation()
override;
162 typename TOutputImage::PixelType m_Min{};
163 typename TOutputImage::PixelType m_Max{};
172 #ifndef ITK_MANUAL_INSTANTIATION
173 # include "itkRandomImageSource.hxx"
ImageBaseType::DirectionType DirectionType
Represent a n-dimensional size (bounds) of a n-dimensional image.
typename TOutputImage::SpacingType SpacingType
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
typename TOutputImage::DirectionType DirectionType
Control indentation during Print() invocation.
typename SizeType::SizeValueType SizeValueType
PointValueType[TOutputImage::ImageDimension] PointValueArrayType
ImageBaseType::IndexType IndexType
Base class for all process objects that output image data.
ImageBaseType::RegionType RegionType
typename TOutputImage::SizeType SizeType
Generate an n-dimensional image of random pixel values.
Define additional traits for native types such as int or float.
typename OutputImageType::RegionType OutputImageRegionType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename OutputImageType::PixelType OutputImagePixelType
SizeValueType[TOutputImage::ImageDimension] SizeValueArrayType
typename TOutputImage::PointValueType PointValueType
SpacingValueType[TOutputImage::ImageDimension] SpacingValueArrayType
typename TOutputImage::IndexType IndexType
typename TOutputImage::SpacingValueType SpacingValueType
unsigned long SizeValueType
typename TOutputImage::PointType PointType