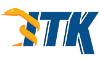 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRecursiveSeparableImageFilter_h
19 #define itkRecursiveSeparableImageFilter_h
51 template <
typename TInputImage,
typename TOutputImage = TInputImage>
88 itkGetConstMacro(Direction,
unsigned int);
91 itkSetMacro(Direction,
unsigned int);
95 SetInputImage(
const TInputImage *);
105 PrintSelf(std::ostream & os,
Indent indent)
const override;
108 BeforeThreadedGenerateData()
override;
111 GenerateData()
override;
125 EnlargeOutputRequestedRegion(
DataObject * output)
override;
177 template <
typename T1,
typename T2>
189 out = a1 * b1 + a2 * b2 + a3 * b3 + a4 * b4;
193 template <
typename T1,
typename T2>
205 const unsigned int sz = a1.
GetSize();
210 for (
unsigned int i = 0; i < sz; ++i)
212 out[i] = a1[i] * b1 + a2[i] * b2 + a3[i] * b3 + a4[i] * b4;
216 template <
typename T1,
typename T2>
228 out -= a1 * b1 + a2 * b2 + a3 * b3 + a4 * b4;
231 template <
typename T1,
typename T2>
243 const unsigned int sz = a1.
GetSize();
248 for (
unsigned int i = 0; i < sz; ++i)
250 out[i] -= a1[i] * b1 + a2[i] * b2 + a3[i] * b3 + a4[i] * b4;
257 unsigned int m_Direction{ 0 };
261 #ifndef ITK_MANUAL_INSTANTIATION
262 # include "itkRecursiveSeparableImageFilter.hxx"
typename NumericTraits< InputPixelType >::ScalarRealType ScalarRealType
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
static void MathEMAMAMAM(VariableLengthVector< T1 > &out, const VariableLengthVector< T1 > &a1, const T2 &b1, const VariableLengthVector< T1 > &a2, const T2 &b2, const VariableLengthVector< T1 > &a3, const T2 &b3, const VariableLengthVector< T1 > &a4, const T2 &b4)
Base class for filters that take an image as input and overwrite that image as the output.
void SetSize(unsigned int sz, TReallocatePolicy reallocatePolicy, TKeepValuesPolicy keepValues)
unsigned int GetSize() const
Base class for recursive convolution with a kernel.
Control indentation during Print() invocation.
Base class for all process objects that output image data.
typename InputImageType::Pointer InputImagePointer
ImageBaseType::RegionType RegionType
TInputImage InputImageType
Represents an array whose length can be defined at run-time.
Define additional traits for native types such as int or float.
typename TInputImage::PixelType InputPixelType
typename OutputImageType::RegionType OutputImageRegionType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static void MathSMAMAMAM(VariableLengthVector< T1 > &out, const VariableLengthVector< T1 > &a1, const T2 &b1, const VariableLengthVector< T1 > &a2, const T2 &b2, const VariableLengthVector< T1 > &a3, const T2 &b3, const VariableLengthVector< T1 > &a4, const T2 &b4)
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
static void MathSMAMAMAM(T1 &out, const T1 &a1, const T2 &b1, const T1 &a2, const T2 &b2, const T1 &a3, const T2 &b3, const T1 &a4, const T2 &b4)
static void MathEMAMAMAM(T1 &out, const T1 &a1, const T2 &b1, const T1 &a2, const T2 &b2, const T1 &a3, const T2 &b3, const T1 &a4, const T2 &b4)
typename InputImageType::ConstPointer InputImageConstPointer
unsigned long SizeValueType
typename NumericTraits< InputPixelType >::RealType RealType
TOutputImage OutputImageType
Base class for all data objects in ITK.