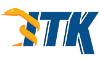 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkShapedNeighborhoodIterator_h
19 #define itkShapedNeighborhoodIterator_h
149 template <
typename TImage,
typename TBoundaryCondition = ZeroFluxNeumannBoundaryCondition<TImage>>
159 static constexpr
unsigned int Dimension = TImage::ImageDimension;
172 using typename Superclass::IndexListType;
173 using typename Superclass::BoundaryConditionType;
174 using typename Superclass::ImageBoundaryConditionPointerType;
175 using typename Superclass::NeighborhoodType;
177 using typename Superclass::ImageType;
193 ConstIterator::operator=(o);
201 ConstIterator::ProtectedSet(v);
207 Iterator(
const Self * s,
const typename IndexListType::const_iterator & li)
229 using Superclass::SetPixel;
230 using Superclass::SetCenterPixel;
237 Superclass::operator=(orig);
247 return Iterator(
this, this->m_ActiveIndexList.begin());
252 return Iterator(
this, this->m_ActiveIndexList.end());
256 using Superclass::Begin;
257 using Superclass::End;
Self & operator=(const Self &orig)
ShapedNeighborhoodIterator(const SizeType &radius, const ImageType *ptr, const RegionType ®ion)
void Set(const PixelType &v) const
Represent a n-dimensional size (bounds) of a n-dimensional image.
typename ImageType ::PixelType PixelType
A light-weight container object for storing an N-dimensional neighborhood of values.
ImageBaseType::SizeType SizeType
typename AllocatorType::const_iterator ConstIterator
A neighborhood iterator which can take on an arbitrary shape.
Iterator(const Self *s, const typename IndexListType::const_iterator &li)
Iterator & operator=(const Iterator &o)
ImageBaseType::IndexType IndexType
typename ImageType ::InternalPixelType InternalPixelType
ImageBaseType::RegionType RegionType
Const version of ShapedNeighborhoodIterator, defining iteration of a local N-dimensional neighborhood...
typename AllocatorType::const_iterator ConstIterator
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
typename OffsetType::OffsetValueType OffsetValueType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Const version of NeighborhoodIterator, defining iteration of a local N-dimensional neighborhood of pi...
typename ImageType ::RegionType RegionType
SizeValueType NeighborIndexType
constexpr unsigned int Dimension
typename AllocatorType::iterator Iterator
unsigned long SizeValueType