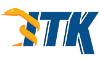 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkSimplexMeshToTriangleMeshFilter_h
19 #define itkSimplexMeshToTriangleMeshFilter_h
42 template <
typename TInputMesh,
typename TOutputMesh>
113 using PointIdIterator =
typename SimplexPolygonType::PointIdIterator;
122 this->m_Mesh->GetPoint(*it, &p);
123 center += p.GetVectorFromOrigin();
131 m_CenterMap->InsertElement(cellId, center);
152 CellInterfaceVisitorImplementation<InputPixelType, InputCellTraitsType, SimplexPolygonType, SimplexCellVisitor>;
163 PrintSelf(std::ostream & os,
Indent indent)
const override;
166 GenerateData()
override;
184 #ifndef ITK_MANUAL_INSTANTIATION
185 # include "itkSimplexMeshToTriangleMeshFilter.hxx"
188 #endif //__SimplexMeshToTriangleMeshFilter_h
SmartPointer< Self > Pointer
typename InputMeshType::CellIdentifier CellIdentifier
SmartPointer< const Self > ConstPointer
void Visit(CellIdentifier cellId, SimplexPolygonType *poly)
visits all polygon cells and compute the cell centers
typename InputMeshType::NeighborListType::iterator InputNeighborsIterator
typename PointMapType::Pointer PointMapPointer
ImageBaseType::PointType PointType
typename InputMeshType::CellType SimplexCellType
typename InputMeshType::NeighborListType InputNeighbors
Represents a polygon in a Mesh.
Convenience class for generating meshes.
Control indentation during Print() invocation.
A wrapper of the STL "map" container.
typename InputPointsContainer::Pointer InputPointsContainerPointer
unsigned int GetNumberOfPoints() const override
typename InputMeshType::PixelType InputPixelType
Light weight base class for most itk classes.
void SetMesh(const InputMeshType *mesh)
This filter converts a 2-simplex mesh into a triangle mesh.
A template class used to implement a visitor object.
PointIdIterator PointIdsEnd() override
typename InputMeshType::PointType InputPointType
typename SimplexVisitorInterfaceType::Pointer SimplexVisitorInterfacePointer
MeshToMeshFilter is the base class for all process objects that output mesh data, and require mesh da...
typename InputMeshType::MeshTraits::CellTraits InputCellTraitsType
typename CellMultiVisitorType::Pointer CellMultiVisitorPointer
typename SimplexCellType::MultiVisitor CellMultiVisitorType
typename InputMeshType::PointsContainer InputPointsContainer
PointMapPointer GetCenterMap()
InputMeshConstPointer m_Mesh
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
PointMapPointer m_CenterMap
typename InputPointsContainer::Iterator InputPointsContainerIterator
PointIdIterator PointIdsBegin() override
typename InputMeshType::PointIdentifier PointIdentifier
typename InputMeshType::ConstPointer InputMeshConstPointer