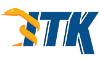 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkTernaryFunctorImageFilter_h
19 #define itkTernaryFunctorImageFilter_h
38 template <
typename TInputImage1,
39 typename TInputImage2,
40 typename TInputImage3,
41 typename TOutputImage,
81 SetInput1(
const TInputImage1 * image1);
85 SetInput2(
const TInputImage2 * image2);
89 SetInput3(
const TInputImage3 * image3);
120 if (!(functor == m_Functor))
129 static constexpr
unsigned int Input1ImageDimension = TInputImage1::ImageDimension;
130 static constexpr
unsigned int Input2ImageDimension = TInputImage2::ImageDimension;
131 static constexpr
unsigned int Input3ImageDimension = TInputImage3::ImageDimension;
132 static constexpr
unsigned int OutputImageDimension = TOutputImage::ImageDimension;
134 #ifdef ITK_USE_CONCEPT_CHECKING
149 BeforeThreadedGenerateData()
override;
170 #ifndef ITK_MANUAL_INSTANTIATION
171 # include "itkTernaryFunctorImageFilter.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
typename Input1ImageType::PixelType Input1ImagePixelType
typename Input2ImageType::PixelType Input2ImagePixelType
typename OutputImageType::Pointer OutputImagePointer
TInputImage2 Input2ImageType
typename Input2ImageType::RegionType Input2ImageRegionType
Base class for filters that take an image as input and overwrite that image as the output.
typename Input2ImageType::ConstPointer Input2ImagePointer
const FunctorType & GetFunctor() const
typename Input3ImageType::PixelType Input3ImagePixelType
Base class for all process objects that output image data.
typename Input3ImageType::RegionType Input3ImageRegionType
FunctorType & GetFunctor()
TInputImage1 Input1ImageType
ImageBaseType::RegionType RegionType
TInputImage3 Input3ImageType
Implements pixel-wise generic operation of three images.
Functor::Add2< typename TInputImage1::PixelType, typename TInputImage2::PixelType, typename TOutputImage::PixelType > FunctorType
typename Input3ImageType::ConstPointer Input3ImagePointer
typename Input1ImageType::ConstPointer Input1ImagePointer
typename OutputImageType::RegionType OutputImageRegionType
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void SetFunctor(const FunctorType &functor)
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename OutputImageType::PixelType OutputImagePixelType
typename Input1ImageType::RegionType Input1ImageRegionType
TOutputImage OutputImageType