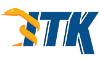 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkTreeContainerBase_h
19 #define itkTreeContainerBase_h
33 template <
typename TValue>
50 SetRoot(
const TValue element) = 0;
66 IsLeaf(
const TValue element) = 0;
70 IsRoot(
const TValue element) = 0;
Represents a node in a tree.
virtual bool IsRoot(const TValue element)=0
virtual bool IsLeaf(const TValue element)=0
void SetSubtree(bool val)
Light weight base class for most itk classes.
A base class for tree containers.
virtual bool Contains(const TValue element)=0
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
virtual bool SetRoot(const TValue element)=0
Base class for most ITK classes.
~TreeContainerBase() override=default
virtual const TreeNode< TValue > * GetRoot() const =0
virtual int Count() const =0