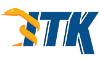 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVXLVideoIO_h
19 #define itkVXLVideoIO_h
22 #ifndef ITK_VIDEO_USE_VXL
23 # define ITK_VIDEO_USE_VXL
27 # pragma warning(disable : 4786)
31 #include "vidl/vidl_ffmpeg_istream.h"
32 #include "vidl/vidl_ffmpeg_ostream.h"
33 #include "vidl/vidl_convert.h"
34 #include "vul/vul_reg_exp.h"
95 Read(
void * buffer)
override;
143 Write(
const void * buffer)
override;
148 const std::vector<SizeValueType> & dim,
150 unsigned int nChannels,
178 vidl_ffmpeg_ostream_params::encoder_type
207 #endif // itkVXLVideoIO_h
TemporalRatioType GetRatio() const override
virtual void SetCameraIndex(int idx)
TemporalRatioType GetFramesPerSecond() const override
void Read(void *buffer) override
void SetReadFromCamera() override
void Write(const void *buffer) override
FrameOffsetType GetLastIFrame() const override
bool CanReadCamera(CameraIDType cameraID) const override
TemporalOffsetType GetPositionInMSec() const override
FrameOffsetType GetFrameTotal() const override
Control indentation during Print() invocation.
void FinishReadingOrWriting() override
void PrintSelf(std::ostream &os, Indent indent) const override
void SetReadFromFile() override
bool CanReadFile(const char *) override
vidl_ffmpeg_ostream_params::encoder_type m_Encoder
vidl_ffmpeg_istream * m_Reader
unsigned int GetSizeFromPixelFormat(vidl_pixel_format fmt)
bool PixelFormatSupported(vidl_pixel_format fmt)
vidl_ffmpeg_ostream_params::encoder_type FourCCtoEncoderType(const char *fourCC)
Light weight base class for most itk classes.
virtual FrameOffsetType GetIFrameInterval() const
void WriteImageInformation() override
void SetWriterParameters(TemporalRatioType fps, const std::vector< SizeValueType > &dim, const char *fourCC, unsigned int nChannels, IOComponentEnum componentType) override
FrameOffsetType GetCurrentFrame() const override
bool SetNextFrameToRead(FrameOffsetType frameNumber) override
double TemporalOffsetType
VideoIO object for reading and writing videos using VXL.
unsigned int GetNChannelsFromPixelFormat(vidl_pixel_format fmt)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
SizeValueType CameraIDType
void UpdateReaderProperties()
vidl_pixel_format m_PixelFormat
SizeValueType FrameOffsetType
Base class for most ITK classes.
vidl_ffmpeg_ostream * m_Writer
Abstract superclass defines video IO interface.
bool CanWriteFile(const char *) override
virtual int GetCameraIndex()
void ReadImageInformation() override
vidl_frame_sptr m_VIDLFrame