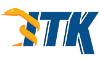 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkWatershedSegmentTable_h
19 #define itkWatershedSegmentTable_h
47 template <
typename TScalar>
79 if (this->height < o.
height)
103 using HashMapType = std::unordered_map<IdentifierType, segment_t>;
107 using DataType =
typename HashMapType::mapped_type;
125 auto result = m_HashMap.find(a);
127 if (result == m_HashMap.end())
133 return &(result->second);
144 if (result == m_HashMap.end())
150 return &(result->second);
159 if (m_HashMap.find(a) == m_HashMap.end())
189 return m_HashMap.empty();
198 typename HashMapType::size_type
201 return m_HashMap.size();
212 return m_HashMap.begin();
220 return m_HashMap.end();
228 return m_HashMap.begin();
236 return m_HashMap.end();
261 return m_MaximumDepth;
270 m_HashMap = o.m_HashMap;
271 m_MaximumDepth = o.m_MaximumDepth;
292 #ifndef ITK_MANUAL_INSTANTIATION
293 # include "itkWatershedSegmentTable.hxx"
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
edge_pair_t(IdentifierType l, ScalarType s)
std::unordered_map< IdentifierType, segment_t > HashMapType
std::list< edge_pair_t > edge_list_t
ScalarType GetMaximumDepth() const
void operator=(const Self &)
HashMapType::size_type Size() const
ConstIterator End() const
typename HashMapType::const_iterator ConstIterator
const segment_t * Lookup(const IdentifierType a) const
typename HashMapType::value_type ValueType
segment_t * Lookup(const IdentifierType a)
void Erase(const IdentifierType a)
class ITK_FORWARD_EXPORT DataObject
typename HashMapType::mapped_type DataType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename HashMapType::iterator Iterator
bool IsEntry(const IdentifierType a) const
unsigned int GetSegmentMemorySize() const
Base class for most ITK classes.
ConstIterator Begin() const
void SetMaximumDepth(ScalarType s)
SizeValueType IdentifierType
Base class for all data objects in ITK.