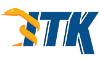 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkZeroFluxNeumannImageNeighborhoodPixelAccessPolicy_h
20 #define itkZeroFluxNeumannImageNeighborhoodPixelAccessPolicy_h
41 template <
typename TImage>
69 return (indexValue <= 0) ? 0
70 : (static_cast<ImageSizeValueType>(indexValue) < imageSizeValue)
72 : static_cast<IndexValueType>(imageSizeValue - 1);
~ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy()=default
Represent a n-dimensional index in a n-dimensional image.
SizeValueType ImageSizeValueType
ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy & operator=(const ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy &)=delete
const IndexValueType m_PixelIndexValue
typename TImage::PixelType PixelType
static IndexValueType CalculatePixelIndexValue(const ImageSizeType &imageSize, const OffsetType &offsetTable, const IndexType &pixelIndex) noexcept
ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy(const ImageSizeType &imageSize, const OffsetType &offsetTable, const NeighborhoodAccessorFunctorType &neighborhoodAccessor, const IndexType &pixelIndex) noexcept
typename TImage::InternalPixelType InternalPixelType
typename TImage::NeighborhoodAccessorFunctorType NeighborhoodAccessorFunctorType
typename TImage::ImageDimensionType ImageDimensionType
PixelType GetPixelValue(const InternalPixelType *const imageBufferPointer) const noexcept
static constexpr ImageDimensionType ImageDimension
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy()=delete
const NeighborhoodAccessorFunctorType & m_NeighborhoodAccessor
void SetPixelValue(InternalPixelType *const imageBufferPointer, const PixelType &pixelValue) const noexcept
unsigned long SizeValueType
static IndexValueType GetClampedIndexValue(const IndexValueType indexValue, const ImageSizeValueType imageSizeValue) noexcept