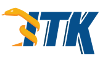 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageIORegion_h
19 #define itkImageIORegion_h
79 GetImageDimension()
const;
85 GetRegionDimension()
const;
89 GetRegionType()
const override;
113 operator=(
const Self & region);
117 operator=(
Self &&) =
default;
127 GetModifiableIndex();
146 GetSize(
unsigned long i)
const;
149 GetIndex(
unsigned long i)
const;
171 IsInside(
const Self & region)
const;
176 GetNumberOfPixels()
const;
184 PrintSelf(std::ostream & os,
Indent indent)
const override;
187 unsigned int m_ImageDimension{ 2 };
194 extern ITKCommon_EXPORT std::ostream &
195 operator<<(std::ostream & os,
const ImageIORegion & region);
203 template <
unsigned int VDimension>
230 const unsigned int imageDimension = VDimension;
232 const unsigned int minDimension = std::min(ioDimension, imageDimension);
237 for (
unsigned int i = 0; i < minDimension; ++i)
239 outIORegion.
SetSize(i, size[i]);
240 outIORegion.
SetIndex(i, index[i] - largestRegionIndex[i]);
246 for (
unsigned int k = minDimension; k < ioDimension; ++k)
276 const unsigned int imageDimension = VDimension;
278 const unsigned int minDimension = std::min(ioDimension, imageDimension);
280 for (
unsigned int i = 0; i < minDimension; ++i)
282 size[i] = inIORegion.
GetSize(i);
283 index[i] = inIORegion.
GetIndex(i) + largestRegionIndex[i];
Size< Self::ImageDimension > SizeType
Index< Self::ImageDimension > IndexType
::itk::OffsetValueType OffsetValueType
void SetIndex(const IndexType &index)
::itk::SizeValueType SizeValueType
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
typename ImageRegionType::IndexType ImageIndexType
std::vector< IndexValueType > IndexType
Helper class for converting ImageRegions into ImageIORegions and back.
const IndexType & GetIndex() const
ImageBaseType::SizeType SizeType
::itk::IndexValueType IndexValueType
const SizeType & GetSize() const
Control indentation during Print() invocation.
An ImageIORegion represents a structured region of data.
std::vector< SizeValueType > SizeType
ImageBaseType::IndexType IndexType
typename ImageRegionType::SizeType ImageSizeType
static void Convert(const ImageRegionType &inImageRegion, ImageIORegionType &outIORegion, const ImageIndexType &largestRegionIndex)
const IndexType & GetIndex() const
A region represents some portion or piece of data.
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
unsigned int GetImageDimension() const
const SizeType & GetSize() const
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
static void Convert(const ImageIORegionType &inIORegion, ImageRegionType &outImageRegion, const ImageIndexType &largestRegionIndex)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
signed long OffsetValueType
void SetIndex(const IndexType &index)
signed long IndexValueType
void SetSize(const SizeType &size)
void SetSize(const SizeType &size)
unsigned long SizeValueType