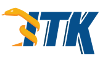 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkImageRegion_h
29 #define itkImageRegion_h
41 template <
unsigned int VImageDimension>
68 template <
unsigned int VImageDimension>
80 static constexpr
unsigned int ImageDimension = VImageDimension;
84 static constexpr
unsigned int SliceDimension = ImageDimension - (ImageDimension > 1);
90 return ImageDimension;
112 return Superclass::RegionEnum::ITK_STRUCTURED_REGION;
149 operator=(
const Self &) ITK_NOEXCEPT =
default;
222 GetUpperIndex()
const;
230 ComputeOffsetTable(OffsetTableType offsetTable)
const;
236 return (m_Index == region.m_Index) && (m_Size == region.m_Size);
243 return !(*
this == region);
250 for (
unsigned int i = 0; i < ImageDimension; i++)
252 if (index[i] < m_Index[i])
256 if (index[i] >= (m_Index[i] + static_cast<IndexValueType>(m_Size[i])))
269 template <
typename TCoordRepType>
273 for (
unsigned int i = 0; i < ImageDimension; i++)
275 if (Math::RoundHalfIntegerUp<IndexValueType>(index[i]) < static_cast<IndexValueType>(m_Index[i]))
280 const auto bound = static_cast<TCoordRepType>(m_Index[i] + m_Size[i] - 0.5);
289 if (!(index[i] <= bound))
304 IndexType beginCorner = region.GetIndex();
306 if (!this->IsInside(beginCorner))
311 const SizeType & size = region.GetSize();
312 for (
unsigned int i = 0; i < ImageDimension; i++)
314 endCorner[i] = beginCorner[i] + static_cast<OffsetValueType>(size[i]) - 1;
316 if (!this->IsInside(endCorner))
326 GetNumberOfPixels()
const;
335 PadByRadius(
const IndexValueArrayType radius);
338 PadByRadius(
const SizeType & radius);
348 ShrinkByRadius(
const IndexValueArrayType radius);
351 ShrinkByRadius(
const SizeType & radius);
358 Crop(
const Self & region);
364 Slice(
const unsigned int dim)
const;
372 PrintSelf(std::ostream & os,
Indent indent)
const override;
382 template <
unsigned int VImageDimension>
387 #ifndef ITK_MANUAL_INSTANTIATION
388 # include "itkImageRegion.hxx"
IndexType & GetModifiableIndex()
typename IndexType::OffsetType OffsetType
typename IndexType::IndexValueType IndexValueType
OffsetValueType[ImageDimension+1] OffsetTableType
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
IndexValueType[ImageDimension] IndexValueArrayType
Base class for templated image classes.
void SetSize(unsigned int i, SizeValueType sze)
const IndexType & GetIndex() const
An image region represents a structured region of data.
ImageBaseType::SizeType SizeType
const SizeType & GetSize() const
class ITK_TEMPLATE_EXPORT ImageBase
Control indentation during Print() invocation.
void SetIndex(unsigned int i, IndexValueType sze)
bool IsInside(const Self ®ion) const
ImageBaseType::IndexType IndexType
bool operator==(const Self ®ion) const noexcept
SizeValueType GetSize(unsigned int i) const
bool IsInside(const IndexType &index) const
bool IsInside(const ContinuousIndex< TCoordRepType, VImageDimension > &index) const
A region represents some portion or piece of data.
static unsigned int GetImageDimension()
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
A templated class holding a point in n-Dimensional image space.
signed long OffsetValueType
Superclass::RegionEnum GetRegionType() const override
void SetIndex(const IndexType &index)
IndexValueType GetIndex(unsigned int i) const
signed long IndexValueType
typename OffsetType::OffsetValueType OffsetValueType
bool operator!=(const Self ®ion) const noexcept
typename SizeType::SizeValueType SizeValueType
void SetSize(const SizeType &size)
SizeType & GetModifiableSize()
unsigned long SizeValueType
ImageRegion(const SizeType &size) noexcept