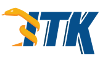 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
38 #include "ITKMeshExport.h"
111 template <
typename TPixelType,
112 unsigned int VDimension = 3,
113 typename TMeshTraits = DefaultStaticMeshTraits<TPixelType, VDimension, VDimension>>
114 class ITK_TEMPLATE_EXPORT
Mesh :
public PointSet<TPixelType, VDimension, TMeshTraits>
117 ITK_DISALLOW_COPY_AND_MOVE(
Mesh);
140 static constexpr
unsigned int PointDimension = TMeshTraits::PointDimension;
141 static constexpr
unsigned int MaxTopologicalDimension = TMeshTraits::MaxTopologicalDimension;
143 #if !defined(ITK_LEGACY_REMOVE)
148 static constexpr CellsAllocationMethodType CellsAllocationMethodUndefined =
149 MeshClassCellsAllocationMethodEnum::CellsAllocationMethodUndefined;
150 static constexpr CellsAllocationMethodType CellsAllocatedAsStaticArray =
152 static constexpr CellsAllocationMethodType CellsAllocatedAsADynamicArray =
154 static constexpr CellsAllocationMethodType CellsAllocatedDynamicallyCellByCell =
229 , m_FeatureId(featureId)
297 GetNumberOfCells()
const;
300 PassStructure(
Self * inputMesh);
303 Initialize()
override;
307 CopyInformation(
const DataObject * data)
override;
315 GetBoundingBox()
const;
328 GetCellLinks()
const;
358 DeleteUnusedCellData();
360 #if !defined(ITK_WRAPPING_PARSER)
374 GetBoundaryAssignments(
int dimension);
377 GetBoundaryAssignments(
int dimension)
const;
410 SetBoundaryAssignment(
int dimension,
424 GetBoundaryAssignment(
int dimension,
434 GetNumberOfCellBoundaryFeatures(
int dimension,
CellIdentifier)
const;
446 GetCellBoundaryFeatureNeighbors(
int dimension,
449 std::set<CellIdentifier> * cellSet);
456 GetCellNeighbors(
CellIdentifier cellId, std::set<CellIdentifier> * cellSet);
471 BuildCellLinks()
const;
492 PrintSelf(std::ostream & os,
Indent indent)
const override;
499 ReleaseCellsMemory();
510 extern ITKMesh_EXPORT std::ostream &
514 #ifndef ITK_MANUAL_INSTANTIATION
515 # ifndef ITK_WRAPPING_PARSER
516 # include "itkMesh.hxx"
typename MeshTraits::PointCellLinksContainer PointCellLinksContainer
typename MeshTraits::CellTraits CellTraits
typename CellType::MultiVisitor CellMultiVisitorType
bool operator==(const Self &r) const
typename CellDataContainer::ConstIterator CellDataContainerIterator
typename PointsContainer::Iterator PointsContainerIterator
typename PointDataContainer::Pointer PointDataContainerPointer
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
A superclass of the N-dimensional mesh structure; supports point (geometric coordinate and attribute)...
typename MeshTraits::CellLinksContainer CellLinksContainer
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
ImageBaseType::PointType PointType
typename BoundingBoxType::Pointer BoundingBoxPointer
CellsContainerPointer m_CellsContainer
typename CellLinksContainer::Pointer CellLinksContainerPointer
typename CellsContainer::Pointer CellsContainerPointer
CellDataContainerPointer m_CellDataContainer
Control indentation during Print() invocation.
A visitor that can visit different cell types in a mesh. CellInterfaceVisitor instances can be regist...
typename CellDataContainer::ConstPointer CellDataContainerConstPointer
A wrapper of the STL "map" container.
typename CellType::CellAutoPointer CellAutoPointer
typename CellLinksContainer::ConstPointer CellLinksContainerConstPointer
typename MeshTraits::CellIdentifier CellIdentifier
MeshClassCellsAllocationMethod
typename MeshTraits::CellsContainer CellsContainer
typename MeshTraits::PointIdentifier PointIdentifier
ImageBaseType::RegionType RegionType
typename CellsContainer::ConstPointer CellsContainerConstPointer
MeshClassCellsAllocationMethodEnum m_CellsAllocationMethod
SelfAutoPointer CellAutoPointer
typename MeshTraits::PixelType PixelType
typename PointsContainer::Pointer PointsContainerPointer
typename MeshTraits::CoordRepType CoordRepType
CellFeatureIdentifier m_FeatureId
typename CellsContainer::ConstIterator CellsContainerConstIterator
typename MeshTraits::InterpolationWeightType InterpolationWeightType
typename PointDataContainer::ConstIterator PointDataContainerIterator
typename MeshTraits::PointsContainer PointsContainer
An abstract interface for cells.
typename MeshTraits::CellFeatureIdentifier CellFeatureIdentifier
typename CellLinksContainer::ConstIterator CellLinksContainerIterator
BoundingBoxPointer m_BoundingBox
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Implements the N-dimensional mesh structure.
typename MeshTraits::CellPixelType CellPixelType
typename MeshTraits::PointDataContainer PointDataContainer
typename PointCellLinksContainer::const_iterator PointCellLinksContainerIterator
Base class for most ITK classes.
std::vector< BoundaryAssignmentsContainerPointer > BoundaryAssignmentsContainerVector
typename CellsContainer::Iterator CellsContainerIterator
typename MeshTraits::PointType PointType
Represent and compute information about bounding boxes.
BoundaryAssignmentsContainerVector m_BoundaryAssignmentsContainers
CellFeatureIdentifier CellFeatureCount
typename PointsContainer::ConstIterator PointsContainerConstIterator
typename CellDataContainer::Pointer CellDataContainerPointer
typename BoundaryAssignmentsContainer::Pointer BoundaryAssignmentsContainerPointer
CellLinksContainerPointer m_CellLinksContainer
BoundaryAssignmentIdentifier(CellIdentifier cellId, CellFeatureIdentifier featureId)
typename MeshTraits::PointHashType PointHashType
typename MeshTraits::CellDataContainer CellDataContainer
Base class for all data objects in ITK.