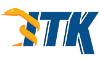 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBSplineControlPointImageFilter_h
19 #define itkBSplineControlPointImageFilter_h
30 #include "vnl/vnl_matrix.h"
31 #include "vnl/vnl_vector.h"
58 template <
typename TInputImage,
typename TOutputImage = TInputImage>
76 static constexpr
unsigned int ImageDimension = TInputImage::ImageDimension;
82 using PixelType =
typename OutputImageType::PixelType;
120 SetSplineOrder(
unsigned int);
131 itkGetConstReferenceMacro(SplineOrder,
ArrayType);
150 itkGetConstReferenceMacro(CloseDimension,
ArrayType);
208 PrintSelf(std::ostream & os,
Indent indent)
const override;
221 BeforeThreadedGenerateData()
override;
248 bool m_DoMultilevel{
false };
249 unsigned int m_MaximumNumberOfLevels{ 1 };
255 vnl_matrix<RealType> m_RefinedLatticeCoefficients[ImageDimension]{};
263 RealType m_BSplineEpsilon{ static_cast<RealType>(1
e-3) };
271 for (
unsigned int i = 1; i < ImageDimension; ++i)
273 k[i] = size[ImageDimension - i - 1] * k[i - 1];
276 for (
unsigned int i = 0; i < ImageDimension; ++i)
278 index[ImageDimension - i - 1] = static_cast<unsigned int>(number / k[ImageDimension - i - 1]);
279 number %= k[ImageDimension - i - 1];
287 #ifndef ITK_MANUAL_INSTANTIATION
288 # include "itkBSplineControlPointImageFilter.hxx"
typename RealImageType::Pointer RealImagePointer
SmartPointer< Self > Pointer
BSpline kernel used for density estimation and nonparametric regression.
ImageBaseType::DirectionType DirectionType
A superclass of the N-dimensional mesh structure; supports point (geometric coordinate and attribute)...
Represent a n-dimensional size (bounds) of a n-dimensional image.
TInputImage ControlPointLatticeType
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
typename OutputImageType::RegionType RegionType
Control indentation during Print() invocation.
typename OutputImageType::PointType PointType
typename OutputImageType::PixelType PixelType
ImageBaseType::IndexType IndexType
Base class for filters that take an image as input and produce an image as output.
Base class for all process objects that output image data.
typename OutputImageType::PointType OriginType
ImageBaseType::RegionType RegionType
RealImageType::IndexType NumberToIndex(unsigned int number, typename RealImageType::SizeType size)
typename MeshTraits::PixelType PixelType
typename PointSetType::PointDataContainer PointDataContainerType
typename OutputImageType::SpacingType SpacingType
typename PointDataImageType::Pointer PointDataImagePointer
typename OutputImageType::IndexType IndexType
typename OutputImageType::RegionType OutputImageRegionType
typename PointSetType::PixelType PointDataType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename MeshTraits::PointDataContainer PointDataContainer
static constexpr double e
typename OutputImageType::SizeType SizeType
Templated n-dimensional image class.
BSpline kernel used for density estimation and nonparametric regression.
typename OutputImageType::DirectionType DirectionType
Process a given a B-spline grid of control points.
TOutputImage OutputImageType