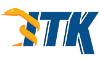 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkConstNeighborhoodIteratorWithOnlyIndex_h
19 #define itkConstNeighborhoodIteratorWithOnlyIndex_h
59 template <
typename TImage>
105 PrintSelf(std::ostream &,
Indent)
const override;
147 return (this->GetIndex() + o);
155 return (this->GetIndex() + this->GetOffset(i));
176 GetBoundingBoxAsImageRegion()
const;
190 Initialize(
const SizeType & radius,
const ImageType * ptr,
const RegionType & region);
197 return (this->GetIndex() == m_BeginIndex);
225 return it.
GetIndex() == this->GetIndex();
228 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
269 this->SetLoop(position);
276 operator+=(
const OffsetType &);
282 operator-=(
const OffsetType &);
309 IndexInBounds(
const NeighborIndexType n, OffsetType & internalIndex, OffsetType & offset)
const;
315 this->SetNeedToUseBoundaryCondition(
true);
321 this->SetNeedToUseBoundaryCondition(
false);
327 m_NeedToUseBoundaryCondition = b;
333 return m_NeedToUseBoundaryCondition;
343 m_IsInBoundsValid =
false;
357 m_BeginIndex = start;
390 mutable bool m_IsInBounds{
false };
395 mutable bool m_IsInBoundsValid{
false };
404 bool m_NeedToUseBoundaryCondition{
false };
407 template <
typename TImage>
408 inline ConstNeighborhoodIteratorWithOnlyIndex<TImage>
417 template <
typename TImage>
418 inline ConstNeighborhoodIteratorWithOnlyIndex<TImage>
425 template <
typename TImage>
426 inline ConstNeighborhoodIteratorWithOnlyIndex<TImage>
436 #ifndef ITK_MANUAL_INSTANTIATION
437 # include "itkConstNeighborhoodIteratorWithOnlyIndex.hxx"
void SetLoop(const IndexType &p)
SmartPointer< const Self > ConstPointer
void SetBeginIndex(const IndexType &start)
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
Represent a n-dimensional size (bounds) of a n-dimensional image.
IndexType GetIndex(NeighborIndexType i) const
RegionType GetRegion() const
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
A light-weight container object for storing an N-dimensional neighborhood of values.
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
OffsetType operator-(const Self &b) const
typename NeighborhoodType::NeighborIndexType NeighborIndexType
const ImageType * GetImagePointer() const
char DummyNeighborhoodPixelType
ImageBaseType::IndexType IndexType
void NeedToUseBoundaryConditionOff()
IndexType GetBeginIndex() const
void SetLocation(const IndexType &position)
ImageBaseType::RegionType RegionType
typename AllocatorType::iterator Iterator
unsigned int DimensionValueType
void NeedToUseBoundaryConditionOn()
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
void SetNeedToUseBoundaryCondition(bool b)
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
typename AllocatorType::const_iterator ConstIterator
bool operator==(const Self &it) const
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
IndexType GetBound() const
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
IndexType GetIndex(const OffsetType &o) const
IndexType GetIndex() const
Index-only version of ConstNeighborhoodIterator, defining iteration of a local N-dimensional neighbor...
SizeValueType NeighborIndexType
typename TImage::RegionType RegionType
constexpr unsigned int Dimension
IndexValueType GetBound(NeighborIndexType n) const
bool GetNeedToUseBoundaryCondition() const