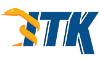 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkDeformableSimplexMesh3DFilter_h
29 #define itkDeformableSimplexMesh3DFilter_h
76 template <
typename TInputMesh,
typename TOutputMesh>
153 itkSetMacro(Iterations,
int);
154 itkGetConstMacro(Iterations,
int);
158 itkSetMacro(Alpha,
double);
159 itkGetConstMacro(Alpha,
double);
163 itkSetMacro(Beta,
double);
164 itkGetConstMacro(Beta,
double);
168 itkSetMacro(Gamma,
double);
169 itkGetConstMacro(Gamma,
double);
173 itkSetMacro(Damping,
double);
174 itkGetConstMacro(Damping,
double);
178 itkSetMacro(Rigidity,
unsigned int);
179 itkGetConstMacro(Rigidity,
unsigned int);
186 itkGetConstMacro(ImageWidth,
int);
187 itkGetConstMacro(ImageHeight,
int);
188 itkGetConstMacro(ImageDepth,
int);
192 itkGetConstMacro(Step,
int);
198 PrintSelf(std::ostream & os,
Indent indent)
const override;
202 GenerateData()
override;
226 ComputeDisplacement();
253 UpdateReferenceMetrics();
259 L_Func(
const double r,
const double d,
const double phi,
double & output);
299 unsigned int m_Rigidity{};
325 #ifndef ITK_MANUAL_INSTANTIATION
326 # include "itkDeformableSimplexMesh3DFilter.hxx"
329 #endif // itkDeformableSimplexMesh3DFilter_h
SmartPointer< Self > Pointer
itk::Point< double, 3 > PointType
typename OutputMeshType::Pointer OutputMeshPointer
typename InputMeshType::Pointer InputMeshPointer
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
handle geometric properties for vertices of a simplex mesh
A wrapper of the STL "map" container.
ImageBaseType::IndexType IndexType
Light weight base class for most itk classes.
MeshToMeshFilter is the base class for all process objects that output mesh data, and require mesh da...
A templated class holding a n-Dimensional covariant vector.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Templated n-dimensional image class.
TOutputMesh OutputMeshType