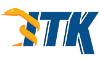 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageIORegion_h
19 #define itkImageIORegion_h
79 GetImageDimension()
const;
85 GetRegionDimension()
const;
89 GetRegionType()
const override;
113 operator=(
const Self & region);
117 operator=(
Self &&) =
default;
127 GetModifiableIndex();
146 GetSize(
unsigned int i)
const;
149 GetIndex(
unsigned int i)
const;
161 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
169 IsInside(
const Self & otherRegion)
const;
174 GetNumberOfPixels()
const;
182 PrintSelf(std::ostream & os,
Indent indent)
const override;
185 unsigned int m_ImageDimension{ 2 };
192 extern ITKCommon_EXPORT std::ostream &
193 operator<<(std::ostream & os,
const ImageIORegion & region);
201 template <
unsigned int VDimension>
228 const unsigned int imageDimension = VDimension;
230 const unsigned int minDimension = std::min(ioDimension, imageDimension);
235 for (
unsigned int i = 0; i < minDimension; ++i)
237 outIORegion.
SetSize(i, size[i]);
238 outIORegion.
SetIndex(i, index[i] - largestRegionIndex[i]);
244 for (
unsigned int k = minDimension; k < ioDimension; ++k)
256 auto size = MakeFilled<ImageSizeType>(1);
271 const unsigned int imageDimension = VDimension;
273 const unsigned int minDimension = std::min(ioDimension, imageDimension);
275 for (
unsigned int i = 0; i < minDimension; ++i)
277 size[i] = inIORegion.
GetSize(i);
278 index[i] = inIORegion.
GetIndex(i) + largestRegionIndex[i];
void SetIndex(const IndexType &index)
typename ImageRegionType::IndexType ImageIndexType
std::vector< IndexValueType > IndexType
Helper class for converting ImageRegions into ImageIORegions and back.
const IndexType & GetIndex() const
ImageBaseType::SizeType SizeType
const SizeType & GetSize() const
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
Control indentation during Print() invocation.
An ImageIORegion represents a structured region of data.
std::vector< SizeValueType > SizeType
Size< VImageDimension > SizeType
ImageBaseType::IndexType IndexType
typename ImageRegionType::SizeType ImageSizeType
static void Convert(const ImageRegionType &inImageRegion, ImageIORegionType &outIORegion, const ImageIndexType &largestRegionIndex)
const IndexType & GetIndex() const
itk::IndexValueType IndexValueType
itk::OffsetValueType OffsetValueType
A region represents some portion or piece of data.
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
unsigned int GetImageDimension() const
const SizeType & GetSize() const
static void Convert(const ImageIORegionType &inIORegion, ImageRegionType &outImageRegion, const ImageIndexType &largestRegionIndex)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void SetIndex(const IndexType &index)
Index< VImageDimension > IndexType
void SetSize(const SizeType &size)
void SetSize(const SizeType &size)
itk::SizeValueType SizeValueType
unsigned long SizeValueType