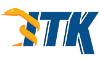 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkImageRegion_h
29 #define itkImageRegion_h
36 #include <type_traits>
42 #ifdef ITK_FUTURE_LEGACY_REMOVE
43 # define itkRegionOverrideMacro // nothing (in the future)
45 # define itkRegionOverrideMacro override
52 template <
unsigned int VImageDimension>
79 template <
unsigned int VImageDimension>
81 #ifndef ITK_FUTURE_LEGACY_REMOVE
90 #ifndef ITK_FUTURE_LEGACY_REMOVE
102 static constexpr
unsigned int ImageDimension = VImageDimension;
106 static constexpr
unsigned int SliceDimension = ImageDimension - (ImageDimension > 1);
112 return ImageDimension;
177 operator=(
const Self &) noexcept =
default;
250 GetUpperIndex()
const;
258 ComputeOffsetTable(OffsetTableType offsetTable)
const;
264 return (m_Index == region.m_Index) && (m_Size == region.m_Size);
267 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
273 for (
unsigned int i = 0; i < ImageDimension; ++i)
275 if (index[i] < m_Index[i] || index[i] >= m_Index[i] + static_cast<IndexValueType>(m_Size[i]))
288 template <
typename TCoordRepType>
292 constexpr TCoordRepType half = 0.5;
293 for (
unsigned int i = 0; i < ImageDimension; ++i)
296 if (!(index[i] >= m_Index[i] - half && index[i] <= (m_Index[i] + static_cast<IndexValueType>(m_Size[i])) - half))
312 const auto & otherIndex = otherRegion.
m_Index;
313 const auto & otherSize = otherRegion.
m_Size;
316 for (
unsigned int i = 0; i < ImageDimension; ++i)
318 if (otherIndex[i] < m_Index[i] || otherSize[i] == 0 ||
319 otherIndex[i] + static_cast<IndexValueType>(otherSize[i]) >
320 m_Index[i] + static_cast<IndexValueType>(m_Size[i]))
331 GetNumberOfPixels()
const;
340 PadByRadius(
const IndexValueArrayType radius);
343 PadByRadius(
const SizeType & radius);
353 ShrinkByRadius(
const IndexValueArrayType radius);
356 ShrinkByRadius(
const SizeType & radius);
363 Crop(
const Self & region);
369 Slice(
const unsigned int dim)
const;
373 template <
size_t VTupleIndex>
377 if constexpr (VTupleIndex == 0)
383 static_assert(VTupleIndex == 1);
390 template <
size_t VTupleIndex>
391 [[nodiscard]]
const auto &
394 if constexpr (VTupleIndex == 0)
400 static_assert(VTupleIndex == 1);
424 template <
unsigned int VImageDimension>
428 template <
unsigned int VImageDimension>
436 #if defined(__clang__)
437 # pragma GCC diagnostic push
440 # pragma GCC diagnostic ignored "-Wmismatched-tags"
454 template <
unsigned int VImageDimension>
455 struct tuple_size<
itk::
ImageRegion<VImageDimension>> : integral_constant<size_t, 2>
459 template <
size_t VTupleIndex,
unsigned int VImageDimension>
461 : conditional<VTupleIndex == 0, itk::Index<VImageDimension>, itk::Size<VImageDimension>>
468 #if defined(__clang__)
469 # pragma GCC diagnostic pop
473 #undef itkRegionOverrideMacro
475 #ifndef ITK_MANUAL_INSTANTIATION
476 # include "itkImageRegion.hxx"
IndexType & GetModifiableIndex()
typename IndexType::OffsetType OffsetType
#define itkRegionOverrideMacro
typename IndexType::IndexValueType IndexValueType
OffsetValueType[ImageDimension+1] OffsetTableType
bool IsInside(const Self &otherRegion) const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
IndexValueType[ImageDimension] IndexValueArrayType
Base class for templated image classes.
void SetSize(unsigned int i, SizeValueType sze)
const IndexType & GetIndex() const
An image region represents a structured region of data.
ImageBaseType::SizeType SizeType
const SizeType & GetSize() const
class ITK_TEMPLATE_EXPORT ImageBase
Control indentation during Print() invocation.
ImageRegion(const Index< VImageDimension > &, const Size< VImageDimension > &) -> ImageRegion< VImageDimension >
void SetIndex(unsigned int i, IndexValueType sze)
ImageBaseType::IndexType IndexType
bool operator==(const Self ®ion) const noexcept
SizeValueType GetSize(unsigned int i) const
bool IsInside(const IndexType &index) const
Region::RegionEnum GetRegionType() const itkRegionOverrideMacro
bool IsInside(const ContinuousIndex< TCoordRepType, VImageDimension > &index) const
A region represents some portion or piece of data.
const char * GetNameOfClass() const itkRegionOverrideMacro
static unsigned int GetImageDimension()
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
A templated class holding a point in n-Dimensional image space.
void SetIndex(const IndexType &index)
IndexValueType GetIndex(unsigned int i) const
typename OffsetType::OffsetValueType OffsetValueType
typename SizeType::SizeValueType SizeValueType
void SetSize(const SizeType &size)
BinaryGeneratorImageFilter< TInputImage1, TInputImage2, TOutputImage > Superclass
SizeType & GetModifiableSize()
unsigned long SizeValueType
ImageRegion(const SizeType &size) noexcept