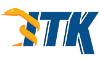 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkPeriodicBoundaryCondition_h
19 #define itkPeriodicBoundaryCondition_h
37 template <
typename TInputImage,
typename TOutputImage = TInputImage>
61 static constexpr
unsigned int ImageDimension = Superclass::ImageDimension;
95 GetInputRequestedRegion(
const RegionType & inputLargestPossibleRegion,
96 const RegionType & outputRequestedRegion)
const override;
107 GetPixel(
const IndexType & index,
const TInputImage * image)
const override;
112 #ifndef ITK_MANUAL_INSTANTIATION
113 # include "itkPeriodicBoundaryCondition.hxx"
typename TOutputImage::PixelType OutputPixelType
Represent a n-dimensional index in a n-dimensional image.
typename TInputImage::PixelType PixelType
An image region represents a structured region of data.
A function object that determines values outside of image boundaries according to periodic (wrap-arou...
A light-weight container object for storing an N-dimensional neighborhood of values.
ImageBaseType::SizeType SizeType
A virtual base object that defines an interface to a class of boundary condition objects for use by n...
typename TInputImage::NeighborhoodAccessorFunctorType NeighborhoodAccessorFunctorType
ImageBaseType::IndexType IndexType
ImageBaseType::RegionType RegionType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TInputImage::InternalPixelType * PixelPointerType