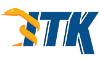 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRayCastInterpolateImageFunction_h
19 #define itkRayCastInterpolateImageFunction_h
40 template <
typename TInputImage,
typename TCoordRep =
double>
53 static constexpr
unsigned int InputImageDimension = TInputImage::ImageDimension;
67 using PixelType =
typename Superclass::InputPixelType;
85 using typename Superclass::OutputType;
88 using typename Superclass::InputImageType;
91 using typename Superclass::RealType;
94 static constexpr
unsigned int ImageDimension = Superclass::ImageDimension;
103 using typename Superclass::ContinuousIndexType;
151 itkSetMacro(Threshold,
double);
152 itkGetConstMacro(Threshold,
double);
182 itkExceptionMacro(
"Input image required!");
184 return input->GetLargestPossibleRegion().GetSize();
192 PrintSelf(std::ostream & os,
Indent indent)
const override;
196 double m_Threshold{};
234 #ifndef ITK_MANUAL_INSTANTIATION
235 # include "itkRayCastInterpolateImageFunction.hxx"
SmartPointer< Self > Pointer
typename TransformType::InputPointType InputPointType
typename InputImageType::SizeType SizeType
Class to hold and manage different parameter types used during optimization.
TInputImage InputImageType
typename Superclass::InputPixelType PixelType
Contains all enum classes used by RayCastHelper class.
typename InputImageType::IndexType IndexType
Projective interpolation of an image at specified positions.
ImageBaseType::PointType PointType
A templated class holding a n-Dimensional vector.
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
NumericTraits< TInputImage::PixelType >::RealType OutputType
ImageBaseType::IndexType IndexType
bool IsInsideBuffer(const PointType &) const override
Light weight base class for most itk classes.
*par Constraints *The filter requires an image with at least two dimensions and a vector *length of at least The theory supports extension to scalar but *the implementation of the itk vector classes do not **The template parameter TRealType must be floating point(float or double) or *a user-defined "real" numerical type with arithmetic operations defined *sufficient to compute derivatives. **\par Performance *This filter will automatically multithread if run with *SetUsePrincipleComponents
typename InterpolatorType::Pointer InterpolatorPointer
bool IsInsideBuffer(const ContinuousIndexType &) const override
typename TransformType::JacobianType TransformJacobianType
bool IsInsideBuffer(const IndexType &) const override
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TransformType::OutputPointType OutputPointType
A templated class holding a geometric point in n-Dimensional space.
typename TransformType::Pointer TransformPointer
Array2D class representing a 2D array.
SizeType GetRadius() const override
Base class for all image interpolators.
typename TransformType::ParametersType TransformParametersType