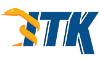 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVectorIndexSelectionCastImageFilter_h
19 #define itkVectorIndexSelectionCastImageFilter_h
27 template <
typename TInput,
typename TOutput>
56 return static_cast<TOutput>(A[
m_Index]);
87 template <
typename TInputImage,
typename TOutputImage>
92 Functor::VectorIndexSelectionCast<typename TInputImage::PixelType, typename TOutputImage::PixelType>>
130 #ifdef ITK_USE_CONCEPT_CHECKING
143 const unsigned int index = this->
GetIndex();
144 const TInputImage * image = this->
GetInput();
146 const unsigned int numberOfRunTimeComponents = image->GetNumberOfComponentsPerPixel();
148 using PixelType =
typename TInputImage::PixelType;
154 const unsigned int numberOfCompileTimeComponents =
sizeof(PixelRealType) /
sizeof(PixelScalarRealType);
156 unsigned int numberOfComponents = numberOfRunTimeComponents;
158 if (numberOfCompileTimeComponents > numberOfRunTimeComponents)
160 numberOfComponents = numberOfCompileTimeComponents;
163 if (index >= numberOfComponents)
165 itkExceptionMacro(
"Selected index = " << index
166 <<
" is greater than the number of components = " << numberOfComponents);
void SetIndex(unsigned int i)
unsigned int GetIndex() const
Implements pixel-wise generic operation on one image.
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(VectorIndexSelectionCast)
VectorIndexSelectionCastImageFilter()=default
Extracts the selected index of the vector that is the input pixel type.
Base class for all process objects that output image data.
void SetIndex(unsigned int i)
void BeforeThreadedGenerateData() override
TOutput operator()(const TInput &A) const
VectorIndexSelectionCast()
Define additional traits for native types such as int or float.
const InputImageType * GetInput() const
bool operator==(const VectorIndexSelectionCast &other) const
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
unsigned int GetIndex() const
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
FunctorType & GetFunctor()
~VectorIndexSelectionCastImageFilter() override=default
virtual void Modified() const
~VectorIndexSelectionCast()=default