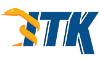 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVoronoiDiagram2D_h
19 #define itkVoronoiDiagram2D_h
50 template <
typename TCoordType>
52 :
public Mesh<TCoordType, 2, DefaultDynamicMeshTraits<TCoordType, 2, 2, TCoordType>>
73 static constexpr
unsigned int PointDimension = MeshTraits::PointDimension;
74 static constexpr
unsigned int MaxTopologicalDimension = MeshTraits::MaxTopologicalDimension;
109 using typename Superclass::CellType;
110 using typename Superclass::CellAutoPointer;
124 itkGetConstMacro(NumberOfSeeds,
unsigned int);
140 NeighborIdsBegin(
int seeds);
143 NeighborIdsEnd(
int seeds);
201 m_CellNeighborsID[x[0]].push_back(x[1]);
202 m_CellNeighborsID[x[1]].push_back(x[0]);
208 m_VoronoiRegions[i]->ClearPoints();
214 m_VoronoiRegions[id]->AddPointId(x);
220 m_VoronoiRegions[id]->BuildEdges();
238 if (this->m_PointsContainer.IsNull())
243 this->m_PointsContainer->Initialize();
249 return static_cast<int>(m_LineList.size());
255 return static_cast<int>(m_EdgeList.size());
261 return static_cast<int>(this->m_PointsContainer->Size());
267 m_LineList.push_back(x);
273 m_EdgeList.push_back(x);
279 this->m_PointsContainer->InsertElement(this->m_PointsContainer->Size(), x);
285 return m_LineList[id];
291 return m_EdgeList[id];
297 return this->m_PointsContainer->ElementAt(
id);
305 x[0] = m_EdgeList[id].m_LeftID;
306 x[1] = m_EdgeList[id].m_RightID;
313 return m_EdgeList[id].m_LineID;
320 PrintSelf(std::ostream & os,
Indent indent)
const override;
324 unsigned int m_NumberOfSeeds{};
325 std::vector<PolygonCellType *> m_VoronoiRegions{};
328 std::vector<std::vector<int>> m_CellNeighborsID{};
330 std::vector<EdgeInfo> m_LineList{};
331 std::vector<VoronoiEdge> m_EdgeList{};
336 #ifndef ITK_MANUAL_INSTANTIATION
337 # include "itkVoronoiDiagram2D.hxx"
void AddCellNeighbor(EdgeInfo x)
typename PointsContainer::ConstIterator PointsContainerConstIterator
SmartPointer< Self > Pointer
typename MeshTraits::CellDataContainer CellDataContainer
Implements the 2-Dimensional Voronoi Diagram.
TInterpolationWeight InterpolationWeightType
void AddEdge(VoronoiEdge x)
itkMakeCellTraitsMacro CellTraits
PointType GetVertex(int id)
std::vector< int > INTvector
typename CellLinksContainer::Pointer CellLinksContainerPointer
IdentifierType PointIdentifier
typename MeshTraits::CoordRepType CoordRepType
ImageBaseType::PointType PointType
typename MeshTraits::PointCellLinksContainer PointCellLinksContainer
typename PointsContainer::Pointer PointsContainerPointer
std::list< PointType > PointList
typename CellsContainer::Pointer CellsContainerPointer
typename CellDataContainer::Pointer CellDataContainerPointer
Represents a polygon in a Mesh.
Control indentation during Print() invocation.
typename MeshTraits::CellsContainer CellsContainer
std::vector< PointType > SeedsType
void VoronoiRegionAddPointId(int id, int x)
typename BoundingBoxType::Pointer BoundingBoxPointer
A visitor that can visit different cell types in a mesh. CellInterfaceVisitor instances can be regist...
typename PointDataContainer::Pointer PointDataContainerPointer
void AddVert(PointType x)
A wrapper of the STL "map" container.
typename CellType::CellAutoPointer CellAutoPointer
typename MeshTraits::CellFeatureIdentifier CellFeatureIdentifier
typename CellDataContainer::ConstIterator CellDataContainerIterator
typename PointCellLinksContainer::const_iterator PointCellLinksContainerIterator
std::deque< EdgeInfo > EdgeInfoDQ
typename CellLinksContainer::ConstIterator CellLinksContainerIterator
VoronoiEdge GetEdge(int id)
typename std::vector< VoronoiEdge >::iterator VoronoiEdgeIterator
typename MeshTraits::PointsContainer PointsContainer
typename CellType::MultiVisitor CellMultiVisitorType
PointsContainerIterator VertexIterator
typename CellsContainer::Iterator CellsContainerIterator
CellFeatureIdentifier CellFeatureCount
typename MeshTraits::PointType PointType
typename CellsContainer::ConstIterator CellsContainerConstIterator
Represents a line segment for a Mesh.
typename MeshTraits::InterpolationWeightType InterpolationWeightType
typename Edge::SelfAutoPointer EdgeAutoPointer
typename MeshTraits::CellAutoPointer genericCellPointer
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename MeshTraits::PointDataContainer PointDataContainer
typename MeshTraits::CellTraits CellTraits
Implements the N-dimensional mesh structure.
typename PointDataContainer::ConstIterator PointDataContainerIterator
EdgeInfo GetEdgeEnd(int id)
Base class for most ITK classes.
IdentifierType CellIdentifier
typename MeshTraits::CellLinksContainer CellLinksContainer
typename MeshTraits::CellIdentifier CellIdentifier
A templated class holding a geometric point in n-Dimensional space.
typename CellType::CellAutoPointer CellAutoPointer
typename PointsContainer::Iterator PointsContainerIterator
std::set< CellIdentifier > PointCellLinksContainer
Represent and compute information about bounding boxes.
typename INTvector::iterator NeighborIdIterator
typename MeshTraits::PixelType PixelType
typename SeedsType::iterator SeedsIterator
A simple structure that holds type information for a mesh and its cells.
int GetEdgeLineID(int id)
Base class for all data objects in ITK.
IdentifierType CellFeatureIdentifier
typename MeshTraits::PointIdentifier PointIdentifier