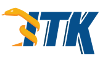 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkImageRegionRange_h
20 #define itkImageRegionRange_h
28 #include <type_traits>
71 template <
typename TImage>
102 template <
bool VIsConst>
138 const SizeType & regionSize) ITK_NOEXCEPT
148 template <std::
size_t VIndex>
151 static_assert(VIndex < (
ImageDimension - 1),
"For a larger index value, the other overload should be picked");
159 this->Increment<VIndex + 1>(std::integral_constant<
bool, (VIndex + 1) < (
ImageDimension - 1)>{});
163 template <std::
size_t VIndex>
166 static_assert(VIndex == (
ImageDimension - 1),
"For a smaller index value, the other overload should be picked");
173 template <std::
size_t VIndex>
176 static_assert(VIndex < (
ImageDimension - 1),
"For a larger index value, the other overload should be picked");
184 this->Decrement<VIndex + 1>(std::integral_constant<
bool, (VIndex + 1) < (
ImageDimension - 1)>{});
188 template <std::
size_t VIndex>
191 static_assert(VIndex == (
ImageDimension - 1),
"For a smaller index value, the other overload should be picked");
201 using value_type =
typename std::iterator_traits<QualifiedBufferIteratorType>::value_type;
202 using reference =
typename std::iterator_traits<QualifiedBufferIteratorType>::reference;
203 using pointer =
typename std::iterator_traits<QualifiedBufferIteratorType>::pointer;
233 this->Increment<0>(std::integral_constant<
bool, (
ImageDimension > 1)>{});
255 this->Decrement<0>(std::integral_constant<
bool, (
ImageDimension > 1)>{});
279 return lhs.m_BufferIterator == rhs.m_BufferIterator;
288 return !(lhs == rhs);
307 bufferedRegionIndex, index, offsetTable.
data(), offsetValue);
349 assert(offsetTable !=
nullptr);
352 if (iterationRegion.GetNumberOfPixels() > 0)
358 const auto & bufferedRegion = image.GetBufferedRegion();
360 itkAssertOrThrowMacro((bufferedRegion.IsInside(iterationRegion)),
361 "Iteration region " << iterationRegion <<
" is outside of buffered region "
409 return this->
begin();
454 return std::accumulate(
465 return sizeValue == 0;
typename TImage::OffsetType OffsetType
~QualifiedIterator()=default
ImageRegionRange(TImage &image)
std::reverse_iterator< iterator > reverse_iterator
std::reverse_iterator< const_iterator > const_reverse_iterator
iterator begin() const noexcept
OffsetTableType m_OffsetTable
typename ImageBufferRange< TImage >::iterator BufferIteratorType
SizeType m_IterationRegionSize
const_iterator cend() const noexcept
typename std::iterator_traits< QualifiedBufferIteratorType >::value_type value_type
typename std::iterator_traits< QualifiedBufferIteratorType >::reference reference
std::ptrdiff_t difference_type
~ImageRegionRange()=default
typename std::conditional< UsingPointerAsIterator, const InternalPixelType *, QualifiedIterator< true > >::type const_iterator
bool empty() const noexcept
ImageBaseType::SizeType SizeType
QualifiedIterator operator++(int) noexcept
QualifiedIterator & operator--() noexcept
typename std::iterator_traits< QualifiedBufferIteratorType >::pointer pointer
ImageBaseType::IndexType IndexType
friend bool operator==(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
void Increment(std::false_type) noexcept
QualifiedIterator(const QualifiedBufferIteratorType &bufferIterator, const OffsetTableType &offsetTable, const OffsetType &iterationOffset, const SizeType ®ionSize) noexcept
QualifiedIterator()=default
void Decrement(std::false_type) noexcept
static constexpr ImageDimensionType ImageDimension
typename std::conditional< VIsConst, typename ImageBufferRange< TImage >::const_iterator, typename ImageBufferRange< TImage >::iterator >::type QualifiedBufferIteratorType
QualifiedIterator operator--(int) noexcept
ImageBaseType::RegionType RegionType
QualifiedIterator(const QualifiedIterator< false > &arg) noexcept
OffsetTableType m_OffsetTable
reverse_iterator rbegin() const noexcept
typename std::conditional< UsingPointerAsIterator, QualifiedInternalPixelType *, QualifiedIterator< IsImageTypeConst > >::type iterator
reverse_iterator rend() const noexcept
QualifiedIterator< IsImageTypeConst > iterator
iterator end() const noexcept
const_reverse_iterator crend() const noexcept
IndexType m_BufferedRegionIndex
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
OffsetType m_IterationOffset
static constexpr bool IsImageTypeConst
signed long OffsetValueType
typename TImage::SizeType SizeType
IndexType m_IterationRegionIndex
typename RegionType::IndexType IndexType
QualifiedBufferIteratorType m_BufferIterator
void Increment(std::true_type) noexcept
reference operator*() const noexcept
const_iterator cbegin() const noexcept
BufferIteratorType m_BufferBegin
const_reverse_iterator crbegin() const noexcept
typename TImage::PixelType PixelType
QualifiedIterator & operator++() noexcept
std::vcl_size_t size() const noexcept
typename TImage::RegionType RegionType
typename TImage::ImageDimensionType ImageDimensionType
QualifiedIterator< true > const_iterator
static OffsetValueType ComputeOffset(const OffsetTableType &offsetTable, const IndexType &bufferedRegionIndex, const IndexType &index)
void Decrement(std::true_type) noexcept
friend bool operator!=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
unsigned long SizeValueType
QualifiedIterator & operator=(const QualifiedIterator &) noexcept=default
static void ComputeOffset(const IndexType &bufferedRegionIndex, const IndexType &index, const OffsetValueType offsetTable[], OffsetValueType &offset)
ImageRegionRange() noexcept=default
SizeType m_IterationRegionSize
std::bidirectional_iterator_tag iterator_category