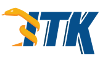 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
24 #include <vxl_version.h>
25 #include "vnl/vnl_matrix_fixed.hxx"
26 #include "vnl/vnl_transpose.h"
27 #include "vnl/algo/vnl_matrix_inverse.h"
28 #include "vnl/vnl_matrix.h"
29 #include "vnl/algo/vnl_determinant.h"
50 template <
typename T,
unsigned int NRows = 3,
unsigned int NColumns = 3>
62 static constexpr
unsigned int RowDimensions = NRows;
63 static constexpr
unsigned int ColumnDimensions = NColumns;
83 vnl_vector_fixed<T, NRows>
operator*(
const vnl_vector_fixed<T, NColumns> & inVNLvect)
const;
88 template <
unsigned int OuterDim>
100 operator+=(
const Self & matrix);
107 operator-=(
const Self & matrix);
110 vnl_matrix<T>
operator*(
const vnl_matrix<T> & matrix)
const;
114 operator*=(
const CompatibleSquareMatrixType & matrix);
118 operator*=(
const vnl_matrix<T> & matrix);
121 vnl_vector<T>
operator*(
const vnl_vector<T> & vc)
const;
160 return m_Matrix(row, col);
167 return m_Matrix(row, col);
171 inline T *
operator[](
unsigned int i) {
return m_Matrix[i]; }
174 inline const T *
operator[](
unsigned int i)
const {
return m_Matrix[i]; }
177 inline InternalMatrixType &
184 inline const InternalMatrixType &
194 m_Matrix.set_identity();
201 m_Matrix.fill(value);
213 inline Matrix(
const vnl_matrix<T> & matrix)
223 for (
unsigned int r = 0; r < NRows; r++)
225 for (
unsigned int c = 0; c < NColumns; c++)
246 this->m_Matrix = matrix;
256 inline vnl_matrix_fixed<T, NColumns, NRows>
261 itkGenericExceptionMacro(<<
"Singular matrix. Determinant is 0.");
263 vnl_matrix_inverse<T> inverse(m_Matrix.as_ref());
264 return vnl_matrix_fixed<T, NColumns, NRows>{ inverse.as_matrix() };
269 inline vnl_matrix_fixed<T, NColumns, NRows>
272 return vnl_matrix_fixed<T, NColumns, NRows>{ m_Matrix.transpose().as_matrix() };
295 template <
typename T,
unsigned int NRows,
unsigned int NColumns>
304 template <
typename T,
unsigned int NRows,
unsigned int NColumns>
313 #ifndef ITK_MANUAL_INSTANTIATION
314 # include "itkMatrix.hxx"
void swap(const Matrix< T, NRows, NColumns > &a, const Matrix< T, NRows, NColumns > &b)
InternalMatrixType & GetVnlMatrix()
void Fill(const T &value)
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
const T * operator[](unsigned int i) const
bool operator!=(const Self &matrix) const
vnl_matrix_fixed< double, NRows, NColumns > InternalMatrixType
void operator*=(const T &value)
InternalMatrixType m_Matrix
void swap(Array< T > &a, Array< T > &b)
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
const InternalMatrixType & GetVnlMatrix() const
const Self & operator=(const InternalMatrixType &matrix)
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
vnl_matrix_fixed< T, NColumns, NRows > GetInverse() const
Matrix< T, NRows, OuterDim > operator*(const vnl_matrix_fixed< T, NRows, OuterDim > &matrix) const
Matrix(const vnl_matrix< T > &matrix)
T * operator[](unsigned int i)
vnl_matrix_fixed< T, NColumns, NRows > GetTranspose() const
Matrix(const InternalMatrixType &matrix)
const Self & operator=(const vnl_matrix< T > &matrix)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
A templated class holding a M x N size Matrix.
CovariantVector< T, NVectorDimension > operator*(const T &scalar, const CovariantVector< T, NVectorDimension > &v)
Define additional traits for native types such as int or float.
A templated class holding a n-Dimensional covariant vector.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void operator/=(const T &value)
Self operator/(const T &value) const
bool operator==(const Self &matrix) const
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Self operator*(const T &value) const
A templated class holding a geometric point in n-Dimensional space.
T & operator()(unsigned int row, unsigned int col)
const T & operator()(unsigned int row, unsigned int col) const