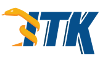 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRGBAPixel_h
19 #define itkRGBAPixel_h
58 template <
typename TComponent =
unsigned short>
70 static constexpr
unsigned int Length = 4;
91 template <
typename TRGBAPixelValueType>
103 operator=(
const ComponentType r[4]);
110 Self
operator*(
const ComponentType & r)
const;
112 operator/(
const ComponentType & r)
const;
117 operator+=(
const Self & r);
119 operator-=(
const Self & r);
121 operator*=(
const ComponentType & r);
123 operator/=(
const ComponentType & r);
144 return this->operator[](c);
151 return static_cast<ComponentType>(
152 std::sqrt(static_cast<double>(this->
operator[](0)) * static_cast<double>(this->
operator[](0)) +
153 static_cast<double>(this->
operator[](1)) * static_cast<double>(this->
operator[](1)) +
154 static_cast<double>(this->
operator[](2)) * static_cast<double>(this->
operator[](2))));
161 this->operator[](c) = v;
168 this->operator[](0) = red;
175 this->operator[](1) = green;
182 this->operator[](2) = blue;
189 this->operator[](3) = alpha;
196 this->operator[](0) = red;
197 this->operator[](1) = green;
198 this->operator[](2) = blue;
199 this->operator[](3) = alpha;
204 const ComponentType &
207 return this->operator[](0);
211 const ComponentType &
214 return this->operator[](1);
218 const ComponentType &
221 return this->operator[](2);
225 const ComponentType &
228 return this->operator[](3);
233 GetLuminance()
const;
236 template <
typename TComponent>
238 operator<<(std::ostream & os,
const RGBAPixel<TComponent> & c);
240 template <
typename TComponent>
242 operator>>(std::istream & is, RGBAPixel<TComponent> & c);
244 template <
typename T>
263 #ifndef ITK_MANUAL_INSTANTIATION
264 # include "itkRGBAPixel.hxx"
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
const ComponentType & GetBlue() const
void SetGreen(ComponentType green)
ComponentType GetScalarValue() const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
RGBAPixel(const RGBAPixel< TRGBAPixelValueType > &r)
void SetNthComponent(int c, const ComponentType &v)
void swap(Array< T > &a, Array< T > &b)
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
RGBAPixel(const ComponentType r[4])
static unsigned int GetNumberOfComponents()
std::istream & operator>>(std::istream &is, Point< T, NPointDimension > &vct)
void SetRed(ComponentType red)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
RGBAPixel(const ComponentType &r)
const ComponentType & GetRed() const
Simulate a standard C array with copy semantics.
CovariantVector< T, NVectorDimension > operator*(const T &scalar, const CovariantVector< T, NVectorDimension > &v)
void Set(ComponentType red, ComponentType green, ComponentType blue, ComponentType alpha)
Represent Red, Green, Blue and Alpha components for color images.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const ComponentType & GetGreen() const
ComponentType GetNthComponent(int c) const
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
const ComponentType & GetAlpha() const
void SetAlpha(ComponentType alpha)
constexpr unsigned int Dimension
void SetBlue(ComponentType blue)
typename NumericTraits< ComponentType >::RealType LuminanceType