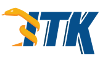 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkShapeLabelObject_h
19 #define itkShapeLabelObject_h
42 template <
typename TLabel,
unsigned int VImageDimension>
166 if (s ==
"NumberOfPixels")
170 else if (s ==
"PhysicalSize")
174 else if (s ==
"Centroid")
178 else if (s ==
"BoundingBox")
182 else if (s ==
"NumberOfPixelsOnBorder")
186 else if (s ==
"PerimeterOnBorder")
190 else if (s ==
"FeretDiameter")
194 else if (s ==
"PrincipalMoments")
198 else if (s ==
"PrincipalAxes")
202 else if (s ==
"Elongation")
206 else if (s ==
"Perimeter")
210 else if (s ==
"Roundness")
214 else if (s ==
"EquivalentSphericalRadius")
218 else if (s ==
"EquivalentSphericalPerimeter")
222 else if (s ==
"EquivalentEllipsoidDiameter")
226 else if (s ==
"Flatness")
230 else if (s ==
"PerimeterOnBorderRatio")
234 else if (s ==
"OrientedBoundingBoxOrigin")
238 else if (s ==
"OrientedBoundingBoxSize")
253 name =
"NumberOfPixels";
256 name =
"PhysicalSize";
262 name =
"BoundingBox";
265 name =
"NumberOfPixelsOnBorder";
268 name =
"PerimeterOnBorder";
271 name =
"FeretDiameter";
274 name =
"PrincipalMoments";
277 name =
"PrincipalAxes";
289 name =
"EquivalentSphericalRadius";
292 name =
"EquivalentSphericalPerimeter";
295 name =
"EquivalentEllipsoidDiameter";
301 name =
"PerimeterOnBorderRatio";
304 name =
"OrientedBoundingBoxOrigin";
307 name =
"OrientedBoundingBoxSize";
637 for (
unsigned int i = 0; i < VImageDimension; i++)
640 for (
unsigned int j = 0; j < VImageDimension; j++)
649 result->SetMatrix(matrix);
650 result->SetOffset(offset);
664 for (
unsigned int i = 0; i < VImageDimension; i++)
667 for (
unsigned int j = 0; j < VImageDimension; j++)
675 result->SetMatrix(matrix);
676 result->SetOffset(offset);
679 result->GetInverse(inverse);
684 template <
typename TSourceLabelObject>
688 Superclass::template CopyAttributesFrom<TSourceLabelObject>(src);
711 template <
typename TSourceLabelObject>
715 itkAssertOrThrowMacro((src !=
nullptr),
"Null Pointer");
716 this->
template CopyLinesFrom<TSourceLabelObject>(src);
717 this->
template CopyAttributesFrom<TSourceLabelObject>(src);
750 os << indent <<
"Perimeter: " <<
m_Perimeter << std::endl;
754 os << indent <<
"Elongation: " <<
m_Elongation << std::endl;
755 os << indent <<
"Flatness: " <<
m_Flatness << std::endl;
756 os << indent <<
"Roundness: " <<
m_Roundness << std::endl;
757 os << indent <<
"Centroid: " <<
m_Centroid << std::endl;
758 os << indent <<
"BoundingBox: ";
static constexpr AttributeType PRINCIPAL_AXES
const RegionType & GetBoundingBox() const
unsigned int AttributeType
Vector< double, VImageDimension > OrientedBoundingBoxSizeType
typename LineType::LengthType LengthType
const VectorType & GetPrincipalMoments() const
static constexpr AttributeType PERIMETER
void CopyAllFrom(const TSourceLabelObject *src)
static constexpr AttributeType ELONGATION
static constexpr AttributeType EQUIVALENT_ELLIPSOID_DIAMETER
static AttributeType GetAttributeFromName(const std::string &s)
void Fill(const T &value)
double m_PerimeterOnBorderRatio
const CentroidType & GetCentroid() const
void SetFlatness(const double &v)
const double & GetFeretDiameter() const
const SizeValueType & GetNumberOfPixelsOnBorder() const
void SetNumberOfPixelsOnBorder(const SizeValueType &v)
const double & GetFlatness() const
An image region represents a structured region of data.
AffineTransformPointer GetPrincipalAxesToPhysicalAxesTransform() const
typename AffineTransformType::Pointer AffineTransformPointer
SizeValueType m_NumberOfPixelsOnBorder
const OrientedBoundingBoxDirectionType & GetOrientedBoundingBoxDirection() const
static constexpr AttributeType EQUIVALENT_SPHERICAL_RADIUS
const SizeValueType & GetNumberOfPixels() const
void SetPerimeterOnBorder(const double &v)
VectorType m_PrincipalMoments
void CopyAttributesFrom(const TSourceLabelObject *src)
const double & GetPerimeterOnBorder() const
static constexpr AttributeType EQUIVALENT_SPHERICAL_PERIMETER
double m_PerimeterOnBorder
const RegionType & GetRegion() const
Control indentation during Print() invocation.
void SetPhysicalSize(const double &v)
const double & GetEquivalentSphericalPerimeter() const
static constexpr AttributeType FERET_DIAMETER
VectorType m_EquivalentEllipsoidDiameter
static constexpr AttributeType CENTROID
void SetEquivalentEllipsoidDiameter(const VectorType &v)
void SetNumberOfPixels(const SizeValueType &v)
Templated n-dimensional image to store labeled objects.
static constexpr AttributeType ORIENTED_BOUNDING_BOX_SIZE
void SetEquivalentSphericalPerimeter(const double &v)
void SetBoundingBox(const RegionType &v)
itk::SizeValueType SizeValueType
static AttributeType GetAttributeFromName(const std::string &s)
const double & GetPerimeterOnBorderRatio() const
Light weight base class for most itk classes.
void SetOrientedBoundingBoxOrigin(const OrientedBoundingBoxPointType &v)
static std::string GetNameFromAttribute(const AttributeType &a)
The base class for the representation of an labeled binary object in an image.
void SetRoundness(const double &v)
void SetFeretDiameter(const double &v)
static constexpr unsigned int ImageDimension
const double & GetElongation() const
static constexpr AttributeType PERIMETER_ON_BORDER_RATIO
void PrintSelf(std::ostream &os, Indent indent) const override
static constexpr AttributeType PERIMETER_ON_BORDER
void SetOrientedBoundingBoxSize(const OrientedBoundingBoxSizeType &v)
void SetPerimeter(const double &v)
const double & GetPerimeter() const
void SetPerimeterOnBorderRatio(const double &v)
Point< double, VImageDimension > OrientedBoundingBoxPointType
SizeValueType m_NumberOfPixels
Simulate a standard C array with copy semantics.
void SetPrincipalMoments(const VectorType &v)
virtual void Print(std::ostream &os, Indent indent=0) const
static constexpr AttributeType BOUNDING_BOX
LabelObjectLine< VImageDimension > LineType
double m_EquivalentSphericalRadius
void SetPrincipalAxes(const MatrixType &v)
void SetCentroid(const CentroidType ¢roid)
static constexpr AttributeType PRINCIPAL_MOMENTS
void SetElongation(const double &v)
double m_EquivalentSphericalPerimeter
Vector< double, VImageDimension > VectorType
Matrix< double, VImageDimension, VImageDimension > MatrixType
const OrientedBoundingBoxSizeType & GetOrientedBoundingBoxSize() const
static constexpr AttributeType ROUNDNESS
Implements a weak reference to an object.
static constexpr AttributeType NUMBER_OF_PIXELS_ON_BORDER
Index< VImageDimension > IndexType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
MatrixType m_PrincipalAxes
static constexpr unsigned int Length
const MatrixType & GetPrincipalAxes() const
A Label object to store the common attributes related to the shape of the object.
ImageRegion< VImageDimension > RegionType
static constexpr AttributeType PHYSICAL_SIZE
AffineTransformPointer GetPhysicalAxesToPrincipalAxesTransform() const
const double & GetRoundness() const
static constexpr AttributeType FLATNESS
const double & GetEquivalentSphericalRadius() const
OrientedBoundingBoxPointType m_OrientedBoundingBoxOrigin
Point< double, VImageDimension > CentroidType
typename Superclass::AttributeType AttributeType
MatrixType OrientedBoundingBoxDirectionType
OrientedBoundingBoxSizeType m_OrientedBoundingBoxSize
void SetEquivalentSphericalRadius(const double &v)
static std::string GetNameFromAttribute(const AttributeType &a)
static constexpr AttributeType ORIENTED_BOUNDING_BOX_ORIGIN
void PrintSelf(std::ostream &os, Indent indent) const override
OrientedBoundingBoxVerticesType GetOrientedBoundingBoxVertices() const
const VectorType & GetEquivalentEllipsoidDiameter() const
static constexpr AttributeType NUMBER_OF_PIXELS
const double & GetPhysicalSize() const
unsigned long SizeValueType
FixedArray< OrientedBoundingBoxPointType, Math::UnsignedPower< unsigned int >(2, ImageDimension)> OrientedBoundingBoxVerticesType
void Fill(const ValueType &)
const OrientedBoundingBoxPointType & GetOrientedBoundingBoxOrigin() const