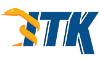 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkExpNegativeImageFilter_h
19 #define itkExpNegativeImageFilter_h
33 template <
typename TInput,
typename TOutput>
53 return static_cast<TOutput>(std::exp(-
m_Factor * static_cast<double>(A)));
87 template <
typename TInputImage,
typename TOutputImage>
92 Functor::ExpNegative<typename TInputImage::PixelType, typename TOutputImage::PixelType>>
129 #ifdef ITK_USE_CONCEPT_CHECKING
Computes the function exp(-K.x) for each input pixel.
Implements pixel-wise generic operation on one image.
ExpNegativeImageFilter()=default
bool ExactlyEquals(const TInput1 &x1, const TInput2 &x2)
Return the result of an exact comparison between two scalar values of potentially different types.
void SetFactor(double factor)
~ExpNegativeImageFilter() override=default
Base class for all process objects that output image data.
void SetFactor(double factor)
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TOutput operator()(const TInput &A) const
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
FunctorType & GetFunctor()
bool operator==(const ExpNegative &other) const
virtual void Modified() const
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(ExpNegative)