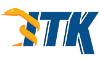 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkQuaternionRigidTransform_h
19 #define itkQuaternionRigidTransform_h
23 #include "vnl/vnl_quaternion.h"
47 template <
typename TParametersValueType =
double>
66 static constexpr
unsigned int InputSpaceDimension = 3;
67 static constexpr
unsigned int OutputSpaceDimension = 3;
68 static constexpr
unsigned int SpaceDimension = 3;
69 static constexpr
unsigned int ParametersDimension = 7;
72 using typename Superclass::ParametersType;
73 using typename Superclass::ParametersValueType;
74 using typename Superclass::FixedParametersType;
75 using typename Superclass::FixedParametersValueType;
117 SetIdentity()
override;
125 SetParameters(
const ParametersType & parameters)
override;
127 const ParametersType &
128 GetParameters()
const override;
136 ComputeJacobianWithRespectToParameters(
const InputPointType & p, JacobianType & jacobian)
const override;
139 #if !defined(ITK_LEGACY_REMOVE)
141 const OutputVectorType & offset);
148 ComputeMatrix()
override;
151 ComputeMatrixParameters()
override;
156 m_Rotation = rotation;
159 const InverseMatrixType &
160 GetInverseMatrix()
const;
163 PrintSelf(std::ostream & os,
Indent indent)
const override;
171 #ifndef ITK_MANUAL_INSTANTIATION
172 # include "itkQuaternionRigidTransform.hxx"
A templated class holding a n-Dimensional vector.
Control indentation during Print() invocation.
A templated class holding a n-Dimensional covariant vector.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
A templated class holding a geometric point in n-Dimensional space.
Array2D class representing a 2D array.