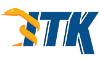 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRigid3DPerspectiveTransform_h
19 #define itkRigid3DPerspectiveTransform_h
22 #include "vnl/vnl_quaternion.h"
37 template <
typename TParametersValueType =
double>
44 static constexpr
unsigned int InputSpaceDimension = 3;
45 static constexpr
unsigned int OutputSpaceDimension = 2;
48 static constexpr
unsigned int SpaceDimension = 3;
49 static constexpr
unsigned int ParametersDimension = 6;
68 using typename Superclass::FixedParametersType;
70 using typename Superclass::ParametersType;
133 SetParameters(
const ParametersType & parameters)
override;
135 const ParametersType &
136 GetParameters()
const override;
157 SetRotation(
const VersorType & rotation);
172 m_FocalDistance = focalDistance;
179 return m_FocalDistance;
186 TransformPoint(
const InputPointType &
point)
const override;
189 using Superclass::TransformVector;
194 itkExceptionMacro(
"TransformVector(const InputVectorType &) is not implemented for Rigid3DPerspectiveTransform");
201 <<
"TransformVector(const InputVnlVectorType &) is not implemented for Rigid3DPerspectiveTransform");
204 using Superclass::TransformCovariantVector;
206 OutputCovariantVectorType
209 itkExceptionMacro(
"TransformCovariantVector(const InputCovariantVectorType &) is not implemented for "
210 "Rigid3DPerspectiveTransform");
217 return m_RotationMatrix;
227 ComputeJacobianWithRespectToParameters(
const InputPointType & p, JacobianType & jacobian)
const override;
232 itkExceptionMacro(
"ComputeJacobianWithRespectToPosition not yet implemented "
236 using Superclass::ComputeJacobianWithRespectToPosition;
239 itkGetConstReferenceMacro(FixedOffset, OffsetType);
240 itkSetMacro(FixedOffset, OffsetType);
244 itkSetMacro(CenterOfRotation, InputPointType);
245 itkGetConstReferenceMacro(CenterOfRotation, InputPointType);
252 PrintSelf(std::ostream & os,
Indent indent)
const override;
262 TParametersValueType m_FocalDistance{};
275 #ifndef ITK_MANUAL_INSTANTIATION
276 # include "itkRigid3DPerspectiveTransform.hxx"
const char * GetNameOfClass() const override
ImageBaseType::SpacingType VectorType
Control indentation during Print() invocation.
TParametersValueType ValueType
*par Constraints *The filter requires an image with at least two dimensions and a vector *length of at least The theory supports extension to scalar but *the implementation of the itk vector classes do not **The template parameter TRealType must be floating point(float or double) or *a user-defined "real" numerical type with arithmetic operations defined *sufficient to compute derivatives. **\par Performance *This filter will automatically multithread if run with *SetUsePrincipleComponents
TParametersValueType ValueType
A templated class holding a n-Dimensional covariant vector.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Array2D class representing a 2D array.