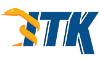 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
22 #include "vnl/vnl_quaternion.h"
23 #include "vnl/vnl_vector_fixed.h"
85 GetVnlQuaternion()
const;
111 Set(T x, T y, T z, T w);
118 operator*=(
const Self & v);
125 operator/=(
const Self & v);
143 GetConjugate()
const;
149 GetReciprocal()
const;
158 operator/(
const Self & v)
const;
165 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
220 Set(
const VectorType & axis, ValueType angle);
228 Set(
const MatrixType & mat);
245 SetRotationAroundX(ValueType angle);
254 SetRotationAroundY(ValueType angle);
263 SetRotationAroundZ(ValueType angle);
279 Transform(
const CovariantVectorType & v)
const;
287 Transform(
const VnlVectorType & v)
const;
301 Exponential(ValueType exponent)
const;
305 static inline ValueType
310 static inline ValueType
315 static inline ValueType
335 template <
typename T>
340 os << v.
GetX() <<
", " << v.
GetY() <<
", ";
341 os << v.
GetZ() <<
", " << v.
GetW() <<
" ]";
345 template <
typename T>
350 #ifndef ITK_MANUAL_INSTANTIATION
351 # include "itkVersor.hxx"
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
vnl_quaternion< TParametersValueType > VnlQuaternionType
static ValueType Epsilon()
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
A templated class holding a unit quaternion.
TParametersValueType ValueType
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
A templated class holding a M x N size Matrix.
A templated class holding a n-Dimensional covariant vector.
static ValueType Epsilon(double *)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static ValueType Epsilon(float *)
static constexpr double e
A templated class holding a geometric point in n-Dimensional space.
vnl_vector_fixed< TParametersValueType, 3 > VnlVectorType
std::istream & operator>>(std::istream &is, Point< T, VPointDimension > &vct)
typename NumericTraits< ValueType >::RealType RealType