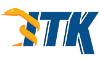 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
24 #include <vxl_version.h>
25 #include "vnl/vnl_matrix_fixed.hxx"
26 #include "vnl/vnl_transpose.h"
27 #include "vnl/algo/vnl_matrix_inverse.h"
28 #include "vnl/vnl_matrix.h"
29 #include "vnl/algo/vnl_determinant.h"
31 #include <type_traits>
51 template <
typename T,
unsigned int VRows = 3,
unsigned int VColumns = 3>
81 static constexpr
unsigned int RowDimensions = VRows;
82 static constexpr
unsigned int ColumnDimensions = VColumns;
102 vnl_vector_fixed<T, VRows>
operator*(
const vnl_vector_fixed<T, VColumns> & inVNLvect)
const;
107 template <
unsigned int OuterDim>
119 operator+=(
const Self & matrix);
126 operator-=(
const Self & matrix);
129 vnl_matrix<T>
operator*(
const vnl_matrix<T> & matrix)
const;
133 operator*=(
const CompatibleSquareMatrixType & matrix);
137 operator*=(
const vnl_matrix<T> & matrix);
140 vnl_vector<T>
operator*(
const vnl_vector<T> & vc)
const;
179 return m_Matrix(row, col);
186 return m_Matrix(row, col);
190 inline T *
operator[](
unsigned int i) {
return m_Matrix[i]; }
193 inline const T *
operator[](
unsigned int i)
const {
return m_Matrix[i]; }
196 inline InternalMatrixType &
203 inline const InternalMatrixType &
213 m_Matrix.set_identity();
221 internalMatrix.set_identity();
222 return Self{ internalMatrix };
230 m_Matrix.fill(value);
243 inline explicit Matrix(
const vnl_matrix<T> & matrix)
251 template <
typename TElement>
252 explicit Matrix(
const TElement (&elements)[VRows][VColumns])
253 : m_Matrix(&elements[0][0])
255 static_assert(std::is_same_v<TElement, T>,
256 "The type of an element should correspond with this itk::Matrix instantiation.");
265 for (
unsigned int r = 0; r < VRows; ++r)
267 for (
unsigned int c = 0; c < VColumns; ++c)
279 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
285 this->m_Matrix = matrix;
295 inline vnl_matrix_fixed<T, VColumns, VRows>
298 if (vnl_determinant(m_Matrix) == T{})
300 itkGenericExceptionMacro(
"Singular matrix. Determinant is 0.");
302 vnl_matrix_inverse<T> inverse(m_Matrix.as_ref());
303 return vnl_matrix_fixed<T, VColumns, VRows>{ inverse.as_matrix() };
308 inline vnl_matrix_fixed<T, VColumns, VRows>
311 return vnl_matrix_fixed<T, VColumns, VRows>{ m_Matrix.transpose().as_matrix() };
323 constexpr
unsigned int
326 return m_Matrix.size();
333 return m_Matrix.begin();
340 return m_Matrix.end();
347 return m_Matrix.begin();
354 return m_Matrix.end();
361 return m_Matrix.begin();
368 return m_Matrix.end();
382 os << indent <<
"Matrix (" << VRows <<
"x" << VColumns <<
")\n";
383 for (
unsigned int r = 0; r < VRows; ++r)
386 for (
unsigned int c = 0; c < VColumns; ++c)
388 os << m_Matrix(r, c) <<
" ";
398 template <
typename T,
unsigned int VRows,
unsigned int VColumns>
407 template <
typename T,
unsigned int VRows,
unsigned int VColumns>
416 #ifndef ITK_MANUAL_INSTANTIATION
417 # include "itkMatrix.hxx"
const ValueType & const_reference
const T & operator()(unsigned int row, unsigned int col) const
const_iterator begin() const
Self & operator=(const vnl_matrix< T > &matrix)
const T * operator[](unsigned int i) const
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
Self operator/(const T &value) const
constexpr unsigned int size() const
void operator/=(const T &value)
vnl_matrix_fixed< double, VRows, VColumns > InternalMatrixType
Self operator*(const T &value) const
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Matrix(const vnl_matrix< T > &matrix)
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
bool operator==(const Self &matrix) const
Control indentation during Print() invocation.
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
const ValueType * const_pointer
const_iterator cbegin() const
static Self GetIdentity()
const_iterator end() const
T & operator()(unsigned int row, unsigned int col)
const InternalMatrixType & GetVnlMatrix() const
InternalMatrixType & GetVnlMatrix()
Matrix< T, VRows, OuterDim > operator*(const vnl_matrix_fixed< T, VRows, OuterDim > &matrix) const
const ValueType * const_iterator
Self & operator=(const InternalMatrixType &matrix)
T * operator[](unsigned int i)
Matrix(const TElement(&elements)[VRows][VColumns])
A templated class holding a M x N size Matrix.
InternalMatrixType m_Matrix
void Fill(const T &value)
A templated class holding a n-Dimensional covariant vector.
void swap(const Matrix< T, VRows, VColumns > &a, const Matrix< T, VRows, VColumns > &b)
void swap(Array< T > &a, Array< T > &b) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void PrintSelf(std::ostream &os, Indent indent) const
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
A templated class holding a geometric point in n-Dimensional space.
void operator*=(const T &value)
vnl_matrix_fixed< T, VColumns, VRows > GetTranspose() const
vnl_matrix_fixed< T, VColumns, VRows > GetInverse() const
const_iterator cend() const
Matrix(const InternalMatrixType &matrix)