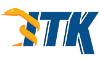 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkTriangleMeshToBinaryImageFilter_h
19 #define itkTriangleMeshToBinaryImageFilter_h
72 template <
typename TInputMesh,
typename TOutputImage>
88 using ValueType =
typename OutputImageType::ValueType;
99 using typename Superclass::OutputImageRegionType;
138 SetSpacing(
const double spacing[3]);
142 SetSpacing(
const float spacing[3]);
159 itkGetConstMacro(InsideValue,
ValueType);
168 itkGetConstMacro(OutsideValue,
ValueType);
177 SetOrigin(
const double origin[3]);
181 SetOrigin(
const float origin[3]);
183 itkGetConstReferenceMacro(Origin,
PointType);
196 using Superclass::SetInput;
203 if (InfoImage != m_InfoImage)
206 m_InfoImage = InfoImage;
215 GetInput(
unsigned int idx);
218 itkSetMacro(Tolerance,
double);
219 itkGetConstMacro(Tolerance,
double);
229 GenerateData()
override;
232 RasterizeTriangles();
236 PolygonToImageRaster(PointVector coords, Point1DArray & zymatrix,
int extent[6]);
248 double m_Tolerance{};
256 PrintSelf(std::ostream & os,
Indent indent)
const override;
260 ComparePoints2D(Point2DType a, Point2DType b);
267 #ifndef ITK_MANUAL_INSTANTIATION
268 # include "itkTriangleMeshToBinaryImageFilter.hxx"
typename OutputImageType::DirectionType DirectionType
SmartPointer< Self > Pointer
Represent a n-dimensional index in a n-dimensional image.
typename InputMeshType::CellType CellType
typename InputMeshType::CellsContainerIterator CellsContainerIterator
ImageBaseType::DirectionType DirectionType
typename InputMeshType::CellsContainerPointer CellsContainerPointer
typename OutputImageType::Pointer OutputImagePointer
A superclass of the N-dimensional mesh structure; supports point (geometric coordinate and attribute)...
Represent a n-dimensional size (bounds) of a n-dimensional image.
typename InputMeshType::PixelType InputPixelType
typename InputMeshType::PointsContainer InputPointsContainer
typename InputPointsContainer::Pointer InputPointsContainerPointer
typename InputMeshType::Pointer InputMeshPointer
ImageBaseType::PointType PointType
typename InputPointsContainer::Iterator InputPointsContainerIterator
typename TOutputImage::SizeType SizeType
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
void SetInfoImage(OutputImageType *InfoImage)
typename OutputImageType::SpacingType SpacingType
std::vector< std::vector< PointType > > PointArray
Point1D(const double p, const int s)
ImageBaseType::IndexType IndexType
Base class for all process objects that output image data.
std::vector< Point2DType > Point2DVector
3D Rasterization algorithm Courtesy of Dr David Gobbi of Atamai Inc.
typename PointSetType::PointsContainer PointsContainer
*par Constraints *The filter requires an image with at least two dimensions and a vector *length of at least The theory supports extension to scalar but *the implementation of the itk vector classes do not **The template parameter TRealType must be floating point(float or double) or *a user-defined "real" numerical type with arithmetic operations defined *sufficient to compute derivatives. **\par Performance *This filter will automatically multithread if run with *SetUsePrincipleComponents
typename OutputImageType::ValueType ValueType
std::vector< PointType > PointVector
TOutputImage OutputImageType
typename MeshTraits::PointsContainer PointsContainer
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
std::vector< std::vector< Point1D > > Point1DArray
std::vector< Point1D > Point1DVector
Point1D(const Point1D &point)
std::vector< std::vector< Point2DType > > Point2DArray
void GenerateOutputInformation() override
typename TOutputImage::IndexType IndexType
typename InputMeshType::PointType InputPointType
typename InputMeshType::MeshTraits::CellTraits InputCellTraitsType