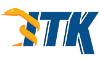 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVersorTransform_h
19 #define itkVersorTransform_h
23 #include "vnl/vnl_quaternion.h"
46 template <
typename TParametersValueType =
double>
65 static constexpr
unsigned int SpaceDimension = 3;
66 static constexpr
unsigned int InputSpaceDimension = 3;
67 static constexpr
unsigned int OutputSpaceDimension = 3;
68 static constexpr
unsigned int ParametersDimension = 3;
72 using typename Superclass::ParametersType;
73 using typename Superclass::FixedParametersType;
109 SetParameters(
const ParametersType & parameters)
override;
112 const ParametersType &
113 GetParameters()
const override;
126 SetIdentity()
override;
138 #if !defined(ITK_LEGACY_REMOVE)
152 m_Versor = newVersor;
157 PrintSelf(std::ostream & os,
Indent indent)
const override;
162 ComputeMatrix()
override;
165 ComputeMatrixParameters()
override;
173 #ifndef ITK_MANUAL_INSTANTIATION
174 # include "itkVersorTransform.hxx"
ImageBaseType::SpacingType VectorType
Control indentation during Print() invocation.
TParametersValueType ValueType
A templated class holding a n-Dimensional covariant vector.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Array2D class representing a 2D array.