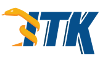 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageAdaptor_h
19 #define itkImageAdaptor_h
26 template <
typename TPixelType,
unsigned int VImageDimension>
55 template <
typename TImage,
typename TAccessor>
65 static constexpr
unsigned int ImageDimension = TImage::ImageDimension;
99 using AccessorFunctorType =
typename InternalImageType::AccessorFunctorType::template Rebind<Self>::Type;
137 template <
typename UPixelType,
unsigned int UImageDimension = TImage::ImageDimension>
143 template <
typename UPixelType,
unsigned int NUImageDimension = TImage::ImageDimension>
154 SetLargestPossibleRegion(
const RegionType & region)
override;
160 SetBufferedRegion(
const RegionType & region)
override;
166 SetRequestedRegion(
const RegionType & region)
override;
173 SetRequestedRegion(
const DataObject * data)
override;
182 GetRequestedRegion()
const override;
193 GetLargestPossibleRegion()
const override;
201 GetBufferedRegion()
const override;
205 Allocate(
bool initialize =
false)
override;
210 Initialize()
override;
216 m_PixelAccessor.Set(m_Image->GetPixel(index), value);
223 return m_PixelAccessor.Get(m_Image->GetPixel(index));
231 GetOffsetTable()
const;
247 return m_Image->GetPixelContainer();
250 const PixelContainer *
253 return m_Image->GetPixelContainer();
259 SetPixelContainer(PixelContainer * container);
272 Graft(
const Self * imgData);
283 GetBufferPointer()
const;
290 SetSpacing(
const double * spacing )
override;
293 SetSpacing(
const float * spacing )
override;
299 GetSpacing()
const override;
305 GetOrigin()
const override;
309 SetOrigin(
const PointType origin)
override;
312 SetOrigin(
const double * origin )
override;
315 SetOrigin(
const float * origin )
override;
325 GetDirection()
const override;
333 Modified()
const override;
337 GetMTime()
const override;
343 return m_PixelAccessor;
350 return m_PixelAccessor;
357 m_PixelAccessor = accessor;
365 CopyInformation(
const DataObject * data)
override;
370 UpdateOutputInformation()
override;
373 SetRequestedRegionToLargestPossibleRegion()
override;
376 PropagateRequestedRegion()
override;
379 UpdateOutputData()
override;
382 VerifyRequestedRegion()
override;
388 template <
typename TCoordRep>
393 return m_Image->TransformPhysicalPointToContinuousIndex(point, index);
400 template <
typename TCoordRep>
404 return m_Image->TransformPhysicalPointToIndex(point, index);
411 template <
typename TCoordRep>
416 m_Image->TransformContinuousIndexToPhysicalPoint(index, point);
424 template <
typename TCoordRep>
428 m_Image->TransformIndexToPhysicalPoint(index, point);
431 template <
typename TCoordRep>
436 m_Image->TransformLocalVectorToPhysicalVector(inputGradient, outputGradient);
439 template <
typename TVector>
443 TVector outputGradient;
444 TransformLocalVectorToPhysicalVector(inputGradient, outputGradient);
445 return outputGradient;
448 template <
typename TCoordRep>
453 m_Image->TransformPhysicalVectorToLocalVector(inputGradient, outputGradient);
456 template <
typename TVector>
460 TVector outputGradient;
461 TransformPhysicalVectorToLocalVector(inputGradient, outputGradient);
462 return outputGradient;
469 PrintSelf(std::ostream & os,
Indent indent)
const override;
472 using Superclass::Graft;
477 template <
typename TPixelType>
479 UpdateAccessor(typename ::itk::VectorImage<TPixelType, ImageDimension> * itkNotUsed(dummy))
481 this->m_PixelAccessor.SetVectorLength(this->m_Image->GetNumberOfComponentsPerPixel());
485 template <
typename T>
500 #ifndef ITK_MANUAL_INSTANTIATION
501 # include "itkImageAdaptor.hxx"
PixelType GetPixel(const IndexType &index) const
typename OffsetType::OffsetValueType OffsetValueType
typename TImage::PixelContainerConstPointer PixelContainerConstPointer
ImageBaseType::DirectionType DirectionType
SizeValueType ModifiedTimeType
Represent a n-dimensional size (bounds) of a n-dimensional image.
typename TImage::PixelContainer PixelContainer
Base class for templated image classes.
AccessorType m_PixelAccessor
An image region represents a structured region of data.
TVector TransformLocalVectorToPhysicalVector(const TVector &inputGradient) const
typename SizeType::SizeValueType SizeValueType
typename Accessor::AddPixelAccessor< TImage::PixelType > ::InternalType InternalPixelType
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
bool TransformPhysicalPointToIndex(const Point< TCoordRep, Self::ImageDimension > &point, IndexType &index) const
void TransformLocalVectorToPhysicalVector(const FixedArray< TCoordRep, Self::ImageDimension > &inputGradient, FixedArray< TCoordRep, Self::ImageDimension > &outputGradient) const
InternalPixelType * InternalPixelPointerType
Control indentation during Print() invocation.
const PixelContainer * GetPixelContainer() const
typename IndexType::IndexValueType IndexValueType
PixelType operator[](const IndexType &index) const
Give access to partial aspects of voxels from an Image.
ImageBaseType::IndexType IndexType
TVector TransformPhysicalVectorToLocalVector(const TVector &inputGradient) const
bool TransformPhysicalPointToContinuousIndex(const Point< TCoordRep, Self::ImageDimension > &point, ContinuousIndex< TCoordRep, Self::ImageDimension > &index) const
Get the continuous index from a physical point.
ImageBaseType::RegionType RegionType
typename InternalImageType::AccessorFunctorType::template Rebind< Self >::Type AccessorFunctorType
void SetPixelAccessor(const AccessorType &accessor)
void TransformIndexToPhysicalPoint(const IndexType &index, Point< TCoordRep, Self::ImageDimension > &point) const
Simulate a standard C array with copy semantics.
typename TImage::PixelContainerPointer PixelContainerPointer
typename Accessor::AddPixelAccessor< TImage::PixelType > ::ExternalType PixelType
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
void TransformPhysicalVectorToLocalVector(const FixedArray< TCoordRep, Self::ImageDimension > &inputGradient, FixedArray< TCoordRep, Self::ImageDimension > &outputGradient) const
void UpdateAccessor(typename ::itk::VectorImage< TPixelType, ImageDimension > *)
Implements a weak reference to an object.
void TransformContinuousIndexToPhysicalPoint(const ContinuousIndex< TCoordRep, Self::ImageDimension > &index, Point< TCoordRep, Self::ImageDimension > &point) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
signed long OffsetValueType
signed long IndexValueType
Base class for most ITK classes.
void SetPixel(const IndexType &index, const PixelType &value)
Templated n-dimensional image class.
AccessorType & GetPixelAccessor()
const AccessorType & GetPixelAccessor() const
PixelContainerPointer GetPixelContainer()
unsigned long SizeValueType
Base class for all data objects in ITK.