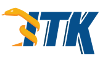 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVectorImage_h
19 #define itkVectorImage_h
80 template <
typename TPixel,
unsigned int VImageDimension = 3>
81 class ITK_TEMPLATE_EXPORT VectorImage :
public ImageBase<VImageDimension>
84 ITK_DISALLOW_COPY_AND_MOVE(VectorImage);
131 static constexpr
unsigned int ImageDimension = VImageDimension;
186 template <
typename UPixelType,
unsigned int NUImageDimension = VImageDimension>
193 template <
typename UElementType,
unsigned int NUImageDimension>
200 template <
typename UPixelType,
unsigned int NUImageDimension = VImageDimension>
206 Allocate(
bool UseDefaultConstructor =
false)
override;
211 Initialize()
override;
226 OffsetValueType offset = m_VectorLength * this->FastComputeOffset(index);
230 (*m_Buffer)[offset + i] = value[i];
242 OffsetValueType offset = m_VectorLength * this->FastComputeOffset(index);
246 return PixelType(&((*m_Buffer)[offset]), m_VectorLength);
261 OffsetValueType offset = m_VectorLength * this->FastComputeOffset(index);
265 return PixelType(&((*m_Buffer)[offset]), m_VectorLength);
290 return m_Buffer ? m_Buffer->GetBufferPointer() :
nullptr;
292 const InternalPixelType *
295 return m_Buffer ? m_Buffer->GetBufferPointer() :
nullptr;
303 return m_Buffer.GetPointer();
307 const PixelContainer *
310 return m_Buffer.GetPointer();
316 SetPixelContainer(PixelContainer * container);
329 Graft(
const Self * image);
346 NeighborhoodAccessorFunctorType
353 const NeighborhoodAccessorFunctorType
360 itkSetMacro(VectorLength, VectorLengthType);
361 itkGetConstReferenceMacro(VectorLength, VectorLengthType);
366 GetNumberOfComponentsPerPixel()
const override;
369 SetNumberOfComponentsPerPixel(
unsigned int n)
override;
374 PrintSelf(std::ostream & os,
Indent indent)
const override;
379 using Superclass::Graft;
390 #ifndef ITK_MANUAL_INSTANTIATION
391 # include "itkVectorImage.hxx"
typename OffsetType::OffsetValueType OffsetValueType
void SetPixel(const IndexType &index, const PixelType &value)
Set a pixel value.
ImageBaseType::DirectionType DirectionType
A structure which enable changing any image class' pixel type to another.
Represent a n-dimensional size (bounds) of a n-dimensional image.
const PixelType GetPixel(const IndexType &index) const
Get a pixel (read only version).
Base class for templated image classes.
An image region represents a structured region of data.
NeighborhoodAccessorFunctorType GetNeighborhoodAccessor()
Templated n-dimensional vector image class.
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
class ITK_TEMPLATE_EXPORT ImageBase
Control indentation during Print() invocation.
const AccessorType GetPixelAccessor() const
const InternalPixelType * GetBufferPointer() const
typename IndexType::IndexValueType IndexValueType
const NeighborhoodAccessorFunctorType GetNeighborhoodAccessor() const
PixelContainer * GetPixelContainer()
TPixelType InternalPixelType
This class provides a common API for pixel accessors for Image and VectorImage. (between the DefaultV...
ImageBaseType::IndexType IndexType
typename PixelContainer::Pointer PixelContainerPointer
Give access to partial aspects of a type.
InternalPixelType * GetBufferPointer()
AccessorType GetPixelAccessor()
const PixelType operator[](const IndexType &index) const
Access a pixel.
ImageBaseType::RegionType RegionType
PixelContainerPointer m_Buffer
typename PixelContainer::ConstPointer PixelContainerConstPointer
Defines an itk::Image front-end to a standard C-array.
typename Rebind< UPixelType, NUImageDimension >::Type RebindImageType
Represents an array whose length can be defined at run-time.
Provides accessor interfaces to Access pixels and is meant to be used on pointers to pixels held by t...
const PixelContainer * GetPixelContainer() const
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
Implements a weak reference to an object.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
signed long OffsetValueType
signed long IndexValueType
Base class for most ITK classes.
PixelType operator[](const IndexType &index)
Access a pixel. This result cannot be used as an lvalue because the pixel is converted on the fly to ...
InternalPixelType IOPixelType
unsigned int VectorLengthType
Base class for all data objects in ITK.
PixelType GetPixel(const IndexType &index)
Get a "reference" to a pixel. This result cannot be used as an lvalue because the pixel is converted ...