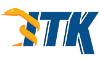 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkLevelSetContainerBase_h
20 #define itkLevelSetContainerBase_h
41 template <
typename TIdentifier,
typename TLevelSet>
95 friend class Iterator;
110 : m_Iterator(it.m_Iterator)
156 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Iterator);
169 return m_Iterator->first;
175 return m_Iterator->second;
194 : m_Iterator(it.m_Iterator)
241 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Iterator);
254 return m_Iterator->first;
260 return m_Iterator->second;
321 HasDomainMap()
const;
331 GetContainer()
const;
342 #ifndef ITK_MANUAL_INSTANTIATION
343 # include "itkLevelSetContainerBase.hxx"
346 #endif // itkLevelSetContainerBase_h
bool operator==(const ConstIterator &it) const
typename HeavisideType::ConstPointer HeavisideConstPointer
SmartPointer< Self > Pointer
LevelSetIdentifierType GetIdentifier() const
SmartPointer< const Self > ConstPointer
Represent a n-dimensional index in a n-dimensional image.
ConstIterator(const LevelSetContainerConstIteratorType &it)
std::map< LevelSetIdentifierType, LevelSetPointer > LevelSetContainerType
typename NumericTraits< OutputType >::RealType OutputRealType
ConstIterator operator++(int)
Represent a n-dimensional size (bounds) of a n-dimensional image.
Base class for the "dense" representation of a level-set function on one image.
std::map< LevelSetIdentifierType, LevelSetDomainType > DomainContainerType
Specifies an image region where an unique std::list of level sets Id's are defined.
LevelSetType * GetLevelSet() const
typename DomainMapImageFilterType::LevelSetDomain LevelSetDomainType
typename DomainMapImageFilterType::Pointer DomainMapImageFilterPointer
std::list< LevelSetIdentifierType > IdListType
ConstIterator(const Iterator &it)
LevelSetType * GetLevelSet() const
ConstIterator & operator++()
ConstIterator & operator*()
Light weight base class for most itk classes.
typename LevelSetType::OutputType OutputType
Base class of the Heaviside function.
bool operator==(const Iterator &it) const
typename LevelSetContainerType::const_iterator LevelSetContainerConstIteratorType
bool operator==(const Iterator &it) const
typename LevelSetType::InputType InputIndexType
A templated class holding a M x N size Matrix.
typename LevelSetType::GradientType GradientType
typename DomainContainerType::iterator DomainIteratorType
A templated class holding a n-Dimensional covariant vector.
ConstIterator & operator--()
TIdentifier LevelSetIdentifierType
typename IdListType::const_iterator IdListConstIterator
typename LevelSetType::HessianType HessianType
typename LevelSetType::OutputRealType OutputRealType
LevelSetContainerIteratorType m_Iterator
LevelSetIdentifierType GetIdentifier() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
std::pair< LevelSetIdentifierType, LevelSetPointer > LevelSetPairType
typename LevelSetContainerType::iterator LevelSetContainerIteratorType
ConstIterator operator--(int)
typename IdListType::iterator IdListIterator
Base class for most ITK classes.
typename LevelSetType::Pointer LevelSetPointer
Templated n-dimensional image class.
TImage::PixelType OutputType
Iterator(const LevelSetContainerIteratorType &it)
ConstIterator * operator->()
constexpr unsigned int Dimension
LevelSetContainerConstIteratorType m_Iterator
bool operator==(const ConstIterator &it) const
Iterator(const ConstIterator &it)
typename LevelSetType::LevelSetDataType LevelSetDataType