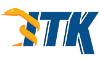 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRGBAPixel_h
19 #define itkRGBAPixel_h
58 template <
typename TComponent =
unsigned short>
70 static constexpr
unsigned int Length = 4;
81 #ifdef ITK_FUTURE_LEGACY_REMOVE
89 template <
typename TRGBAPixelValueType>
98 #if defined(ITK_LEGACY_REMOVE)
105 RGBAPixel(
const ComponentType & r) { this->Fill(r); }
111 operator=(
const ComponentType r[4]);
120 operator/(
const ComponentType & r)
const;
125 operator+=(
const Self & r);
127 operator-=(
const Self & r);
129 operator*=(
const ComponentType & r);
131 operator/=(
const ComponentType & r);
152 return this->operator[](c);
159 return static_cast<ComponentType>(
160 std::sqrt(static_cast<double>(this->
operator[](0)) * static_cast<double>(this->
operator[](0)) +
161 static_cast<double>(this->
operator[](1)) * static_cast<double>(this->
operator[](1)) +
162 static_cast<double>(this->
operator[](2)) * static_cast<double>(this->
operator[](2))));
169 this->operator[](c) = v;
176 this->operator[](0) = red;
183 this->operator[](1) = green;
190 this->operator[](2) = blue;
197 this->operator[](3) = alpha;
204 this->operator[](0) = red;
205 this->operator[](1) = green;
206 this->operator[](2) = blue;
207 this->operator[](3) = alpha;
212 const ComponentType &
215 return this->operator[](0);
219 const ComponentType &
222 return this->operator[](1);
226 const ComponentType &
229 return this->operator[](2);
233 const ComponentType &
236 return this->operator[](3);
241 GetLuminance()
const;
244 template <
typename TComponent>
246 operator<<(std::ostream & os,
const RGBAPixel<TComponent> & c);
248 template <
typename TComponent>
250 operator>>(std::istream & is, RGBAPixel<TComponent> & c);
252 template <
typename T>
271 #ifndef ITK_MANUAL_INSTANTIATION
272 # include "itkRGBAPixel.hxx"
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
const ComponentType & GetBlue() const
void SetGreen(ComponentType green)
ComponentType GetScalarValue() const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
RGBAPixel(const RGBAPixel< TRGBAPixelValueType > &r)
void SetNthComponent(int c, const ComponentType &v)
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
RGBAPixel(const ComponentType r[4])
static unsigned int GetNumberOfComponents()
void SetRed(ComponentType red)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
RGBAPixel(const ComponentType &r)
const ComponentType & GetRed() const
Simulate a standard C array with copy semantics.
void Set(ComponentType red, ComponentType green, ComponentType blue, ComponentType alpha)
Represent Red, Green, Blue and Alpha components for color images.
void swap(Array< T > &a, Array< T > &b) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const ComponentType & GetGreen() const
ComponentType GetNthComponent(int c) const
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
const ComponentType & GetAlpha() const
void SetAlpha(ComponentType alpha)
constexpr unsigned int Dimension
void SetBlue(ComponentType blue)
std::istream & operator>>(std::istream &is, Point< T, VPointDimension > &vct)
typename NumericTraits< ComponentType >::RealType LuminanceType