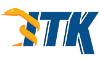 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVariableSizeMatrix_h
19 #define itkVariableSizeMatrix_h
23 #include "vnl/vnl_matrix_fixed.h"
24 #include "vnl/algo/vnl_matrix_inverse.h"
25 #include "vnl/vnl_transpose.h"
26 #include "vnl/vnl_matrix.h"
69 operator+=(
const Self & matrix);
76 operator-=(
const Self & matrix);
83 vnl_matrix<T>
operator*(
const vnl_matrix<T> & matrix)
const;
87 operator*=(
const Self & matrix);
91 operator*=(
const vnl_matrix<T> & matrix);
94 vnl_vector<T>
operator*(
const vnl_vector<T> & vc)
const;
133 return m_Matrix(row, col);
140 return m_Matrix(row, col);
144 inline T *
operator[](
unsigned int i) {
return m_Matrix[i]; }
147 inline const T *
operator[](
unsigned int i)
const {
return m_Matrix[i]; }
150 inline InternalMatrixType &
157 inline const InternalMatrixType &
167 m_Matrix.set_identity();
174 m_Matrix.fill(value);
189 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
203 return vnl_matrix_inverse<T>(m_Matrix).as_matrix();
210 return m_Matrix.transpose();
222 : m_Matrix(matrix.m_Matrix)
229 return m_Matrix.rows();
236 return m_Matrix.cols();
243 return m_Matrix.set_size(r, c);
250 template <
typename T>
261 template <
typename T>
265 if ((matrix.
Rows() != this->Rows()) || (matrix.
Cols() != this->Cols()))
272 for (
unsigned int r = 0; r < this->Rows(); ++r)
274 for (
unsigned int c = 0; c < this->Cols(); ++c)
287 #ifndef ITK_MANUAL_INSTANTIATION
288 # include "itkVariableSizeMatrix.hxx"
Self & operator=(const vnl_matrix< T > &matrix)
bool SetSize(unsigned int r, unsigned int c)
VariableSizeMatrix(const Self &matrix)
T & operator()(unsigned int row, unsigned int col)
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
Self & operator=(const Self &matrix)
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
T * operator[](unsigned int i)
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
void operator/=(const T &value)
A templated class holding a M x N size Matrix.
Self operator/(const T &value) const
vnl_matrix< T > GetInverse() const
const InternalMatrixType & GetVnlMatrix() const
const T * operator[](unsigned int i) const
InternalMatrixType m_Matrix
Self operator*(const T &value) const
InternalMatrixType & GetVnlMatrix()
unsigned int Cols() const
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
vnl_matrix< double > InternalMatrixType
vnl_matrix< T > GetTranspose() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const T & operator()(unsigned int row, unsigned int col) const
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Array class with size defined at construction time.
void Fill(const T &value)
unsigned int Rows() const
void operator*=(const T &value)
bool operator==(const Self &matrix) const