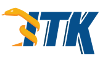 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkStatisticsLabelObject_h
19 #define itkStatisticsLabelObject_h
40 template <
typename TLabel,
unsigned int VImageDimension>
107 else if (s ==
"Maximum")
111 else if (s ==
"Mean")
119 else if (s ==
"StandardDeviation")
123 else if (s ==
"Variance")
127 else if (s ==
"Median")
131 else if (s ==
"MaximumIndex")
135 else if (s ==
"MinimumIndex")
139 else if (s ==
"CenterOfGravity")
149 else if (s ==
"WeightedPrincipalMoments")
153 else if (s ==
"WeightedPrincipalAxes")
157 else if (s ==
"Kurtosis")
161 else if (s ==
"Skewness")
165 else if (s ==
"WeightedElongation")
169 else if (s ==
"Histogram")
173 else if (s ==
"WeightedFlatness")
199 return "StandardDeviation";
208 return "MaximumIndex";
211 return "MinimumIndex";
214 return "CenterOfGravity";
220 return "WeightedPrincipalMoments";
223 return "WeightedPrincipalAxes";
232 return "WeightedElongation";
238 return "WeightedFlatness";
249 template <
typename TSourceLabelObject>
253 Superclass::template CopyAttributesFrom<TSourceLabelObject>(src);
258 m_Sum = src->GetSum();
275 template <
typename TSourceLabelObject>
279 itkAssertOrThrowMacro((src !=
nullptr),
"Null Pointer");
280 this->
template CopyLinesFrom<TSourceLabelObject>(src);
281 this->
template CopyAttributesFrom<TSourceLabelObject>(src);
524 result->SetMatrix(matrix);
525 result->SetOffset(offset);
550 result->SetMatrix(matrix);
551 result->SetOffset(offset);
554 result->GetInverse(inverse);
587 os << indent <<
"Minimum: " <<
m_Minimum << std::endl;
588 os << indent <<
"Maximum: " <<
m_Maximum << std::endl;
589 os << indent <<
"Mean: " <<
m_Mean << std::endl;
590 os << indent <<
"Sum: " <<
m_Sum << std::endl;
592 os << indent <<
"Variance: " <<
m_Variance << std::endl;
593 os << indent <<
"Median: " <<
m_Median << std::endl;
594 os << indent <<
"Skewness: " <<
m_Skewness << std::endl;
595 os << indent <<
"Kurtosis: " <<
m_Kurtosis << std::endl;
604 itkPrintSelfObjectMacro(Histogram);
const double & GetKurtosis() const
typename Superclass::LengthType LengthType
typename LineType::LengthType LengthType
void SetWeightedPrincipalAxes(const MatrixType &v)
static constexpr unsigned int ImageDimension
const MatrixType & GetWeightedPrincipalAxes() const
static constexpr AttributeType SKEWNESS
void SetHistogram(const HistogramType *v)
void Fill(const T &value)
void SetKurtosis(const double &v)
void SetSum(const double &v)
static AttributeType GetAttributeFromName(const std::string &s)
AffineTransformPointer GetPhysicalAxesToWeightedPrincipalAxesTransform() const
An image region represents a structured region of data.
static constexpr AttributeType MEDIAN
typename AffineTransformType::Pointer AffineTransformPointer
static constexpr AttributeType MAXIMUM
A Label object to store the common attributes related to the statistics of the object.
void SetWeightedElongation(const double &v)
static constexpr AttributeType WEIGHTED_PRINCIPAL_MOMENTS
static constexpr AttributeType WEIGHTED_FLATNESS
void SetMaximum(const double &v)
void CopyAttributesFrom(const TSourceLabelObject *src)
double m_StandardDeviation
void SetMinimum(const double &v)
Control indentation during Print() invocation.
static constexpr AttributeType WEIGHTED_ELONGATION
static constexpr AttributeType MEAN
static constexpr AttributeType MINIMUM
void PrintSelf(std::ostream &os, Indent indent) const override
typename Superclass::LineType LineType
void Fill(IndexValueType value)
Matrix< double, Self::ImageDimension, Self::ImageDimension > MatrixType
MatrixType m_WeightedPrincipalAxes
const IndexType & GetMaximumIndex() const
Templated n-dimensional image to store labeled objects.
PointType m_CenterOfGravity
static constexpr AttributeType WEIGHTED_PRINCIPAL_AXES
const double & GetStandardDeviation() const
static AttributeType GetAttributeFromName(const std::string &s)
This class stores measurement vectors in the context of n-dimensional histogram.
const VectorType & GetWeightedPrincipalMoments() const
void SetWeightedFlatness(const double &v)
double m_WeightedFlatness
Light weight base class for most itk classes.
typename Superclass::IndexType IndexType
const double & GetWeightedElongation() const
static constexpr AttributeType STANDARD_DEVIATION
static std::string GetNameFromAttribute(const AttributeType &a)
The base class for the representation of an labeled binary object in an image.
Statistics::Histogram< double > HistogramType
const PointType & GetCenterOfGravity() const
const double & GetMaximum() const
void SetMedian(const double &v)
void SetSkewness(const double &v)
const double & GetMinimum() const
const double & GetMedian() const
const HistogramType * GetHistogram() const
void SetWeightedPrincipalMoments(const VectorType &v)
static constexpr AttributeType KURTOSIS
Vector< double, Self::ImageDimension > VectorType
void SetMean(const double &v)
typename AffineTransformType::Pointer AffineTransformPointer
const double & GetSum() const
const double & GetVariance() const
void SetMinimumIndex(const IndexType &v)
typename Superclass::LabelObjectType LabelObjectType
const double & GetWeightedFlatness() const
typename Superclass::IndexType IndexType
double m_WeightedElongation
void SetStandardDeviation(const double &v)
static constexpr AttributeType MINIMUM_INDEX
static constexpr AttributeType HISTOGRAM
Implements a weak reference to an object.
static constexpr AttributeType SUM
AffineTransformPointer GetWeightedPrincipalAxesToPhysicalAxesTransform() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Point< double, Self::ImageDimension > PointType
VectorType m_WeightedPrincipalMoments
A Label object to store the common attributes related to the shape of the object.
static constexpr AttributeType VARIANCE
const double & GetMean() const
static constexpr AttributeType MAXIMUM_INDEX
static std::string GetNameFromAttribute(const AttributeType &a)
typename Superclass::AttributeType AttributeType
const IndexType & GetMinimumIndex() const
void SetVariance(const double &v)
void SetMaximumIndex(const IndexType &v)
Point< double, VImageDimension > CentroidType
typename Superclass::AttributeType AttributeType
void CopyAllFrom(const TSourceLabelObject *src)
static constexpr AttributeType CENTER_OF_GRAVITY
HistogramType::ConstPointer m_Histogram
void PrintSelf(std::ostream &os, Indent indent) const override
const double & GetSkewness() const
void SetCenterOfGravity(const PointType &v)
void Fill(const ValueType &)